はじめに
この初心者向けガイドでは、AutoML について紹介します。AutoML とカスタム トレーニングの主な違いについては、トレーニング方法の選択をご覧ください。
たとえば、
- あなたがサッカーチームのコーチであったり、
- デジタル小売店のマーケティング部門で働いていたり、
- 建築様式を調べる建築プロジェクトに携わっていたり、
- 自社のウェブサイトに問い合わせフォームがあるとします。
動画、画像、テキスト、テーブルを手動で整理する作業は面倒で、時間もかかります。手動で整理するよりも、コンテンツを自動的に識別してフラグを立てるよう、コンピュータに学習させるほうが楽だと思いませんか。
画像
あなたは建築管理協会に所属し、一貫した建築様式が維持されている地域を特定しようとしています。何十万件もの住宅のスナップショットをふるいにかける必要があります。それらすべての画像を手作業で分類しようとすると、手間がかかりミスも起こりやすくなります。数か月前にインターンが数百枚の画像にラベルを付けましたが、それ以降は誰もデータを確認していません。この作業を代行する方法をコンピュータに教えられるとしたら、とても便利でしょう。
表形式
デジタル小売店のマーケティング部門で働いているとします。あなたとチームメンバーは、顧客のペルソナに基づいてカスタマイズされたメール プログラムを作成しています。ペルソナとマーケティング関連メールの作成が終わり、送信できるようになりました。次は、顧客を小売の好みや消費行動に基づいて各ペルソナに振り分けるシステムを作成する必要があります。このシステムは新しい顧客も対象にします。また、顧客エンゲージメントを最大化するには、メールの送信タイミングを最適化できるように、顧客の消費習慣を予測することも必要になります。
デジタル小売店であれば、顧客とその購入情報に関するデータを持っています。しかし、新しい顧客についてはどうでしょうか。従来の方法では、購入履歴が長い既存顧客であればこうした値を計算できますが、履歴データが少ない顧客の場合はうまくいきません。これらの値を予測するシステムを作ることができ、個人の好みに合ったマーケティング プログラムをすべての顧客に迅速に提供できるとしたらどうでしょうか。
こうした問題の解決にうってつけなのが ML と Vertex AI です。
テキスト
自社のウェブサイトに問い合わせフォームがあるとします。このフォームから毎日多くのメッセージを受け取り、その多くはなんらかの方法で対応可能ですが、すべてのメッセージが一斉に到着するため、メッセージへの対応が遅れがちです。また、異なる従業員がさまざまなメッセージ タイプを扱っています。自動化されたシステムによってメッセージを分類し、適切な担当者が適切なコメントを見られるようになれば、非常に効率的です。
コメントを確認して、それが苦情、過去のサービスに対する褒め言葉、自社ビジネスに対する問い合わせ、面会の予約、関係構築の試みのどれを表しているかを判定するには、システムが必要です。
動画
試合の動画ライブラリが大量にあり、それを分析に使用する場合を考えます。動画を分析に使用したいのですが、レビュー対象の動画は何百時間にも及びます。動画を 1 つずつ確認して、各アクションを区切るために手作業でセグメントにマーク付けする作業は、面倒で、時間もかかります。また、シーズンごとにこの作業を繰り返す必要があります。ここで頭を切り替えて、これらのアクションが動画に表示されるたびに自動的に識別してフラグを付けるコンピュータ モデルを想像してみてください。
目的別のシナリオをいくつかご紹介します。
- 動作認識: ゴールによる得点、ファウルの原因、ペナルティ キックなどに該当するアクションを見つけます。チームの強みと弱みを研究する際に役立ちます。
- 分類: 各動画ショットを、ハーフタイム、試合視点、視聴者視点、コーチ視点などに分類します。関心のある動画だけを閲覧する場合に便利です。
- オブジェクト トラッキング: サッカーボールまたは選手を追跡します。フィールド上のヒートマップ、パス成功率など、選手に関する統計データを取得する場合に便利です。
このガイドでは、AutoML のデータセットとモデルに対して Vertex AI がどのように機能するかについて説明します。また、Vertex AI でどのような問題を解決できるかについても説明します。
公平性に関する注記
Google は、責任ある AI への取り組みの進歩に取り組んでいます。そのため、AutoML を含む ML プロダクトは、公平性や人間中心の ML などの主要な原則を中心に設計されています。独自の ML システムを構築する際のバイアスを軽減するためのベスト プラクティスについては、インクルーシブ ML ガイド - AutoML をご覧ください。
Vertex AI がこの問題に適したツールである理由
従来型のプログラミングでは、コンピュータで扱う手順をプログラマが 1 つずつ指示する必要があります。しかし、サッカーの試合で特定のアクションを識別させるユースケースではどうでしょうか。色、視点、解像度、照明など、考慮すべき要素は多岐にわたるため、コーディングによってマシンに正しい判断を指示するには、ルールの数が多すぎます。どこから手を付ければよいか想像もつかないほどです。また、お客様のコメントは語彙と構造が非常に広範で多様であるため、簡単なルールセットでは捕捉できません。手動でフィルタを作成しようとしても、お客様のコメントの大部分を分類できないことがすぐにわかります。ここで必要になるのが、多様なコメントに対して汎用化できるシステムです。一連の特定のルールが指数関数的に拡大することが想定される状況では、サンプルから学習できるシステムも必要です。
こうした問題の解決に最適なものが ML です。
Vertex AI の仕組み
Vertex AI には、目的に合った結果を得るために使用できる、教師あり学習タスクが含まれています。アルゴリズムやトレーニング方法の詳細は、データの種類とユースケースによって異なります。ML にはさまざまなサブカテゴリがあり、それらはすべて異なる問題を解決し、異なる制約の中で動作します。
画像
分類のためにラベルでアノテーションされたサンプル画像や、オブジェクト検出のためにラベルと境界ボックスの両方でアノテーションされたサンプル画像を使用して、ML モデルのトレーニング、テスト、検証を行います。教師あり学習を使用することで、関心のあるパターンやコンテンツを画像で認識するようモデルをトレーニングできます。
表形式
サンプルデータを使用して ML モデルをトレーニングします。Vertex AI は表形式の(構造化された)データを使用して ML モデルをトレーニングし、新しいデータに対する予測を行います。データセット内の列の 1 つはターゲットと呼ばれ、モデルはそれを学習して予測します。他のデータ列の一部は入力(特徴と呼ばれる)であり、モデルはそこからパターンを学習します。同じ入力特徴を使用してターゲット列とトレーニング オプションを変更するだけで、複数種類のモデルを構築できます。このメール マーケティングの例は、同じ入力特徴でターゲット予測が異なるモデルを構築できることを示しています。あるモデルではお客様のペルソナ(カテゴリ型のターゲット)を予測し、別のモデルでは毎月の支出(数値型のターゲット)を予測します。さらに別のモデルでは、今後 3 か月間の商品の日々の需要(一連の数値型ターゲット)を予測します。
テキスト
Vertex AI を使用すると、教師あり学習を実施できます。これには、ラベル付きデータからパターンを認識するようコンピュータをトレーニングすることが含まれます。教師あり学習を使用することで、関心のあるコンテンツをテキストで認識するよう AutoML モデルをトレーニングできます。
動画
あらかじめラベルが付けられた動画を使用して、ML モデルをトレーニング、テスト、検証します。トレーニング済みのモデルがあれば、新しい動画をモデルに入力し、モデルからラベル付きの動画セグメントを出力できます。動画セグメントにより、動画内の開始時間と終了時間のオフセットが定義されます。この動画セグメントは、動画全体、ユーザー定義の時間セグメント、自動的に検出された動画ショット、または開始時間と終了時間が同じタイムスタンプである可能性があります。ラベルとは、モデルによって予測された「解答」のことです。たとえば、前述のサッカーの例では、新しいサッカーの動画ごとに、モデルタイプに応じて次のように処理されます。
- トレーニング済みの動作認識モデルは、「goal」「personal foul」などのアクション ショットを記述するラベルを含む動画時間オフセットを出力します。
- トレーニング済みの分類モデルは、「game view」「audience view」などのユーザー定義のラベルを含む自動検出されたショット セグメントを出力します。
- トレーニング済みのオブジェクト トラッキング モデルは、オブジェクトが表示されるフレームの境界ボックスを使用してサッカーボールやプレーヤーの軌跡を出力します。
Vertex AI ワークフロー
Vertex AI は、次の標準的な ML ワークフローに従います。
- データの収集: 達成したい結果に向けて、モデルのトレーニング用データとテスト用データを選定します。
- データの準備: データを適切にフォーマットし、ラベルを付けます。
- トレーニング: パラメータを設定してモデルを構築します。
- 評価: モデルの指標を確認します。
- デプロイと予測: モデルを利用できるようにします。
ただし、実際にデータを収集する前に、解決しようとしている問題について検討して、データの要件を把握しておく必要があります。
データの準備
ユースケースを評価する
まずは問題に目を向けて、達成する必要のある結果を考えてください。
画像
データセットを用意するときは、常にユースケースの検討から始めます。まずは以下の点を考慮します。
- どのような結果を得ようとしているか。
- その結果を得るために、どのようなカテゴリまたはオブジェクトを認識する必要があるか。
- 人間がそれらのカテゴリを認識することは可能か。Vertex AI は人間が一度に覚えて割り当てるよりも多くのカテゴリを処理できますが、人間が認識できないカテゴリについては、Vertex AI にとっても認識するのが困難です。
- システムに分類させようとしているデータの種類と範囲を最もよく反映するサンプルはどのようなものか。
表形式
ターゲット列はどのような種類のデータですか。アクセスできるデータはどのくらいありますか。ユーザーが目的とする結果に基づいて、Vertex AI はユースケースを解決するために必要なモデルを作成します。
- バイナリ分類モデルは、2 択の結果(2 つのクラスのうちのいずれか)を予測します。これは、お客様がサブスクリプションを購入するかしないかの予測など、「はい」または「いいえ」の質問に使用します。他の条件がすべて同じ場合、バイナリ分類の問題は他のモデルタイプより必要とするデータが少なくなります。
- 多クラス分類モデルは、1 つのクラスを 3 つ以上の別個のクラスから予測します。これは、ものごとを分類するために使用します。前述の小売店の例では、顧客をさまざまなペルソナに分割するための多クラス分類モデルを構築できます。
- 予測モデルでは、一連の値が予測されます。たとえば、小売店では、商品の在庫を前もって適切に確保できるように、今後 3 か月にわたる商品の日々の需要を予測することが考えられます。
- 回帰モデルは連続値を予測します。小売店の例では、お客様が来月支払う金額を予測する回帰モデルを構築する必要があるでしょう。
テキスト
データセットを用意するときは、常にユースケースの検討から始めます。まずは以下の点を考慮します。
- どのような結果を得ようとしているのか。
- 結果を得るには、どのようなカテゴリを認識する必要があるか。
- 人間がそれらのカテゴリを認識することは可能か。Vertex AI は人間が一度に覚えて割り当てるよりも多くのカテゴリを処理できますが、人間が認識できないカテゴリについては、Vertex AI にとっても認識するのが困難です。
- システムに分類させるデータの種類と範囲を最もよく反映するサンプルはどのようなものか。
動画
実現しようとしている成果に応じて、適切なモデルの目的を選択します。
- 動画の中でゴールによる得点、ファウルの原因、ペナルティ キックなどに該当するアクションを検出するには、動作認識の目的を使用します。
- テレビの映像をコマーシャル、ニュース、テレビ番組などに分類するには、分類の目的を使用します。
- 動画内のオブジェクトの位置を確認して追跡するには、オブジェクト トラッキングの目的を使用します。
動作認識、分類、オブジェクト トラッキングの目的のためのデータセットを準備する際のベスト プラクティスについては、動画データの準備をご覧ください。
データの収集
ユースケースを確立したら、目的のモデルを作成するために使用されるデータを収集します。
画像
必要なデータが決まったら、次にデータの調達方法を見つける必要があります。まずは、組織で現在収集しているあらゆるデータを考慮に入れるところから始めます。モデルのトレーニングに必要なデータがすでに収集されている場合もあります。そのようなデータがない場合、自力で収集することも、サードパーティ プロバイダにデータの収集を委託することもできます。
十分な数のラベル付きサンプルを各カテゴリに含める
Vertex AI Training では、分類のために、カテゴリまたはラベルごとに最低でも 100 個の画像サンプルが必要になります。各ラベルに対応する高品質なサンプルを数多く用意すれば、ラベルがうまく認識される可能性もそれだけ高まります。一般的には、トレーニング プロセスに取り込むラベル付きデータが多いほど、モデル品質は向上します。1 ラベルあたり 1,000 件以上のサンプルを目標にしてください。
カテゴリごとに均等にサンプルを分布させる
カテゴリごとにほぼ同じ数量のトレーニング サンプルを取り込むことが重要です。1 つのラベルに対して豊富なデータがある場合でも、各ラベルの分布を均等にするのがベストです。たとえば、モデルの構築に使用する画像の 80% がモダン様式の一戸建て住宅の写真だとします。このようにラベルの分布が不均等な場合、モデルはあえて危険を冒して一般的でないラベルを予測するよりも、常にモダン様式の一戸建て住宅の写真だと報告する方が安全だと学習する可能性がかなり高くなります。これは、多肢選択式テストを作るときにほとんどすべての正解を「C」にするようなものです。抜け目のない受験生なら、質問を見なくても毎回「C」と答えればよいことにすぐに気付くでしょう。
もちろん各ラベルにほぼ同数のサンプルを調達することは、常に可能であるとは限りません。カテゴリによっては、高品質でバイアスのないサンプルを調達することが困難な場合もあります。そのような状況では、だいたいの目安として、最もサンプル数の少ないラベルが、最もサンプル数の多いラベルの 10% 以上になるようにします。したがって、最もサンプル数の多いラベルに 10,000 個のサンプルがある場合、最もサンプル数の少ないラベルには少なくとも 1,000 個のサンプルが必要になります。
問題空間内のバリエーションを収集する
同様の理由で、問題空間内の多種多様なデータを集めるようにしてください。モデルのトレーニング プロセスで体験する種類が多ければ多いほど、新しいサンプルを一般化しやすくなります。たとえば、家電製品の写真をカテゴリに分類しようとしている場合、トレーニングでモデルに使用する家電製品の種類が多ければ多いほど、見たことのない新機種のタブレット、スマートフォン、ノートパソコンなどを区別できる可能性が高まります。
モデルの意図する出力にデータを合わせる
予測の対象になるものと視覚的に似ている画像を探します。たとえば、冬の雪の日に撮影されたさまざまな家の画像を分類する場合、晴れた日に撮影された家の画像だけでトレーニングされたモデルを使うと、明暗と風景があまりに違いすぎるため、関心のある分類であらかじめタグ付けしたとしても、おそらく良いパフォーマンスは得られないでしょう。トレーニングのサンプルは、そのモデルを使用して分類する予定のものと同じデータセットから作成された実世界のデータにするのが理想的です。
表形式
ユースケースを確立したら、モデルをトレーニングするためのデータを収集する必要があります。データの調達と準備は、ML モデルを構築するうえで重要なステップです。利用できるデータによって、解決できる問題の種類が定まります。使用可能なデータ量はどれくらいですか。それらのデータは、答えを得ようとしている質問の内容と関連があるものですか。データを収集する際には、次の点に注意してください。
関連する特徴を選択する
特徴は、モデルのトレーニングに使用される入力属性です。特徴とは、モデルがパターンを特定して予測を行う方法であるため、問題に関連している必要があります。たとえば、クレジット カード取引が不正であるかどうかを予測するモデルを構築するには、購入者、販売者、金額、日時、購入した商品などの取引詳細を含むデータセットを構築する必要があります。その他に役立つ特徴として、購入者と販売者に関する過去の情報、購入された商品がどのくらいの頻度で不正行為に関係していたかなどが挙げられます。他にはどのような特徴が関連している可能性があるでしょうか。
冒頭の小売店のメール マーケティングのユースケースを考えてみます。必要となる特徴の列の例を以下に示します。
- 購入された商品のリスト(ブランド、カテゴリ、価格、割引など)
- 購入された商品の数(最終日、週、月、年)
- 消費された金額(最終日、週、月、年)
- 商品ごとに、毎日の合計販売数
- 商品ごとに、毎日の合計在庫数
- 特定の日に販促を実施するかどうか
- 既知の買い物客層
十分なデータを含める
一般的に、トレーニング サンプルが多ければ多いほど、成果は上がります。必要なサンプルデータの量は、解決しようとしている問題の複雑さにも比例します。バイナリ分類モデルの場合、正確なモデルを取得するために必要なデータは、マルチクラス モデルと比較するとそれほど多くはありません。なぜなら、1 つのクラスを 2 つのクラスから予測するのは、多数から予測するほど複雑でないからです。
完璧な数式はありませんが、推奨されるサンプルデータの最低数量は次のとおりです。
- 分類問題: 50 行 × 特徴数
- 予測問題:
- 5,000 行 × 特徴数
- 時系列 ID 列に一意の値 10 個 × 特徴数
- 回帰問題: 特徴数 × 200
バリエーションを考慮する
データセットには、問題空間に多様性を持たせてください。モデルのトレーニングで使用するサンプルが多様であればあるほど、目新しいサンプルや一般的ではないサンプルにも対応できる汎用化されたモデルが実現されます。小売店のモデルが、冬季の購入データだけを使ってトレーニングされた場合を想像してみましょう。夏服の嗜好や購入行動をうまく予測することができるでしょうか。
テキスト
必要なデータが決まったら、データの調達方法を見つける必要があります。まずは、組織で現在収集しているあらゆるデータを考慮に入れるところから始めます。モデルをトレーニングするために必要なデータがすでに収集されている場合もあります。必要なデータがない場合、自力で収集することも、サードパーティ プロバイダにデータの収集を委託することもできます。
十分な数のラベル付きサンプルを各カテゴリに含める
各ラベルに対応する高品質なサンプルを数多く用意すれば、ラベルがうまく認識される可能性もそれだけ高まります。一般的には、トレーニング プロセスに取り込むラベル付きデータが多いほど、モデル品質は向上します。必要なサンプルの数は、予測するデータの一貫性の度合いや、目標とする精度によっても異なります。一貫性のあるデータセットのサンプルを少なくするか、精度を 97% ではなく 80% にすることもできます。モデルをトレーニングし、結果を評価します。サンプルを追加して、精度の目標に達するまで再トレーニングします。ラベルごとに数百、数千ものサンプルが必要になることもあります。データ要件と推奨事項の詳細については、AutoML モデル用のテキスト トレーニング データの準備をご覧ください。
カテゴリごとに均等にサンプルを分布させる
カテゴリごとに取得するトレーニング サンプルの数をほぼ同じにすることが重要です。1 つのラベルに対して豊富なデータがある場合でも、各ラベルの分布を均等にするのがベストです。モデルの構築に使用するお客様のコメントの 80% が見積もり依頼だった場合を想像してみてください。このようにラベルの分布が均等でない場合、モデルはあえてあまり一般的でないラベルを予測するよりも、お客様のコメントは見積もり依頼である、と常に報告する方が安全だと学習する可能性が非常に高まります。これは、多肢選択式テストを作るときにほとんどすべての正解を「C」にするようなものです。抜け目のない受験生なら、質問を見なくても毎回「C」と答えればよいことにすぐに気付くでしょう。
各ラベルにほぼ同数のサンプルを調達することは、常に可能であるとは限りません。カテゴリによっては、高品質でバイアスのないサンプルを調達することが困難な場合もあります。そのような状況では、最もサンプル数の少ないラベルが、最もサンプル数の多いラベルの 10% 以上になるようにします。したがって、最もサンプル数の多いラベルに 10,000 個のサンプルがある場合、最もサンプル数の少ないラベルには少なくとも 1,000 個のサンプルが必要になります。
問題空間内のバリエーションを収集する
同様の理由で、問題空間内の多種多様性を反映したデータを集めるようにしてください。提供するサンプルの幅が広くなるほど、モデルは新しいデータをより適切に一般化できます。たとえば、家電製品に関する記事をトピックに分類しようとしているとします。提供されるブランド名と技術仕様が多くなるほど、モデルは記事のトピックをより簡単に判断できるようになります。その記事がトレーニング セットに含まれていないブランドに関するものであっても問題ありません。また、モデルのパフォーマンスをさらに向上させるために、「none_of_the_above」ラベルを含めて、定義されたラベルのいずれとも一致しないドキュメントをこのラベルに分類することもできます。
モデルの意図する出力にデータを合わせる
予測の対象に似たテキストのサンプルを探します。たとえば、ガラス細工に関するソーシャル メディアの投稿を分類しようとしている場合、ガラス細工に関する情報のウェブサイトでトレーニングされたモデルでは、おそらく良いパフォーマンスは得られないでしょう。こうしたウェブサイトでは、語彙やスタイルが異なる可能性があるためです。トレーニングのサンプルは、そのモデルを使用して分類する予定のものと同じデータセットから作成された実世界のデータにするのが理想的です。
動画
ユースケースを確立したら、必要なモデルを作成するために使用する動画データを収集します。トレーニング用に収集するデータによって、解決できる問題の種類が定まります。使用できる動画の数はいくつですか。それらの動画には、モデルが予測するために十分な数のサンプルが含まれていますか。動画データを収集する場合は、次の点を考慮してください。
十分な動画を含める
一般に、データセットに含まれるトレーニング用の動画数が多いほど、正確な結果が得られます。どれくらいの数の動画を収集すればよいかは、解決しようとしている問題の複雑さによっても増減します。たとえば、分類の場合、バイナリ分類問題(2 つのクラスのうちのいずれかを予測)に必要な動画データの量は、マルチラベル分類問題(多数のクラスから 1 つ以上のクラスを予測)の場合よりも少なくなります。
また、実現しようとしている目的の複雑さによっても、必要な動画データの量は変わってきます。サッカーの分類のユースケースでアクション ショットを区別するモデルを構築する場合と、異なる水泳スタイルを分類できるモデルをトレーニングする場合を考えてみましょう。たとえば、平泳ぎ、バタフライ、背泳ぎなどを区別するには、異なる水泳スタイルを識別するためのより多くのトレーニング データが必要になります。モデルに、それぞれのタイプを正確に識別する方法を学習させるためです。動作認識、分類、オブジェクト トラッキングに必要な動画データの最小要件については、動画データの準備をご覧ください。
必要な動画データの量が、手元にある動画より多い場合もあります。その場合は、サードパーティ プロバイダから追加の動画を入手することを検討してください。たとえば、試合のアクション識別モデルに必要な動画が不足している場合は、ハミングバードの動画を購入または入手するという選択肢もあります。
クラス間で均等に動画を配分する
各クラスに同等の数のトレーニング サンプルを用意するようにしてください。たとえば、トレーニング データセットに含まれるサッカー動画のうちの 80% がゴールショットを映したもので、パーソナル ファウルやペナルティ キックの動画は 20% しかない場合を考えてみてください。このような不均等なクラスの配分だと、モデルが特定のアクションをゴールであると予測する確率が高くなります。これは、正解の 80% が「C」である多肢選択式テストを作成するのと似ています。「機転が利く」モデルならば、ほとんどの回答が「C」であることを突き止めてしまいます。
各クラスに同等の数の動画を調達できないことがあります。クラスによっては、高品質でバイアスのないサンプルを用意するのが難しい場合もあります。そのような場合は、1:10 の比率に従うようにしてください。つまり、サンプル数が最も多いクラスに 10,000 本の動画があるとしたら、サンプル数が最も少ないクラスには、少なくとも 1,000 本の動画を用意します。
バリエーションを考慮する
動画データには、問題空間に多様性を持たせてください。モデルのトレーニングで使用するサンプルが多様であればあるほど、目新しいサンプルや一般的ではないサンプルにも対応できる汎用化されたモデルが実現されます。たとえば、サッカーのアクション分類モデルでは、サンプルにさまざまなカメラアングル、日中と夜間、選手の多様な動きを含める必要があります。モデルを多様なデータに触れさせることで、モデルがアクションを区別する能力が高まります。
意図する出力にデータを合わせる

予測対象としてモデルに入力する予定の動画と視覚的に似ているトレーニング用動画を見つけます。たとえば、トレーニング用動画のすべてが冬または夜間に撮影されたものである場合、こうした環境での照明と色のパターンがモデルに影響を与えます。このモデルを使用して夏または日中に撮影された動画をテストすると、正確な予測は得られないでしょう。
次の要素も検討してください。動画の解像度、1 秒あたりの動画フレーム数、カメラアングル、背景
データを準備する
画像
正しい方法(手動またはデフォルト分割)を決定したら、次のいずれかの方法で Vertex AI にデータを追加できます。
- パソコンまたは Cloud Storage から、ラベル(必要な場合は境界ボックスも)を埋め込んだ CSV または JSON Lines 形式のデータをインポートできます。インポート ファイル形式の詳細については、トレーニング データの準備をご覧ください。データセットを手動で分割する場合は、CSV または JSON Lines インポート ファイルで分割を指定できます。
- データにアノテーションが付いていない場合は、ラベルの付いていない画像をアップロードし、Google Cloud コンソールを使用してアノテーションを適用できます。これらのアノテーションは、同じ画像セットに対して複数のアノテーション セットで管理できます。たとえば、単一の画像セットに対して、境界ボックスとラベル情報を含む 1 つのアノテーションを用意してオブジェクト検出を行い、ラベル アノテーションのみを含む別のアノテーション セットを用意して分類を行うことができます。
表形式
利用可能なデータが見つかったら、トレーニングの準備ができていることを確認する必要があります。データに偏りがある場合、または欠損値やエラー値が含まれている場合は、モデルの品質に影響します。モデルのトレーニングを始める前に、次のことを考慮してください。詳細については、こちらをご覧ください。
データの漏出とトレーニング / サービング スキューを防ぐ
データの漏出とは、トレーニング時に入力特徴を使用した際に、予測しようとしているターゲットに関する情報、つまり実際にモデルを使用する際には把握できない情報を「漏出」させることです。これは、ターゲット列との相関性が高い特徴が入力特徴の 1 つとして含まれているときに検出できます。たとえば、顧客が来月にかけてサブスクリプションに申し込むかどうかを予測するモデルを構築しているとします。そして入力特徴の 1 つが、その顧客からの将来のサブスクリプションの支払いであったとします。この場合、テスト時のモデル パフォーマンスは強力になるかもしれませんが、本番環境でデプロイされたときはそうなりません。なぜなら将来のサブスクリプションの支払い情報はサービス提供時に存在しないからです。
トレーニング / サービング スキューとは、トレーニング時に使用される入力特徴が、サービス提供時にモデルに提供される特徴と異なるため、本番環境でモデル品質低下が生じる状態を指します。たとえば、毎時の気温を予測するモデルを構築する際に、一週間ごとの気温のみが含まれるデータを使ってトレーニングした場合です。別の例としては、学生の落第を予測する際に、トレーニング データには学生の成績が常に提供されているが、サービス提供時にはこの情報が提供されない場合です。
トレーニング データを把握することは、データの漏出やトレーニング / サービング スキューを防ぐために重要です。
- データを使用する前に、そのデータが何を意味するか、そのデータを特徴として使用するべきかどうかを必ず把握しておいてください。
- 相関関係を [トレーニング] タブで確認します。相関関係が高い場合は、フラグを付けて見直せるようにしてください。
- トレーニング / サービング スキュー: サービス提供時にまったく同じ形式で使用できる入力特徴だけをモデルに提供するようにしてください。
欠損データ、不完全データ、矛盾するデータをクリーンアップする
サンプルデータの値が欠損していることや正確でないことがよくあります。トレーニングに使用する前に時間をとって見直し、可能であればデータ品質を向上させてください。欠損値が多いほど、データが ML モデルのトレーニングに役立つ度合いが減ってしまいます。
- データに欠損値がないか確認し、可能であれば修正するか、列が null 可能に設定されている場合は、値を空白にします。Vertex AI は欠損値を処理できますが、すべての値を使用できる場合は最適な結果が得られる可能性が高くなります。
- 予測を行うには、トレーニングする行の間隔にばらつきがないことを確認します。Vertex AI は欠損値に代入法で対応できますが、すべての行が使用可能であれば、最適な結果が得られる可能性が高くなります。
- データのエラーやノイズを修正または削除して、データをクリーニングします。一貫性のあるデータにするために、スペル、略語、形式を確認します。
データをインポート後に分析する
Vertex AI には、データセットのインポート後の概要が表示されます。インポートしたデータセットをレビューして、各列の変数型が正しいことを確認してください。Vertex AI は列の値に基づいて変数型を自動的に検出しますが、それぞれを手動で確認することをおすすめします。さらに、null 値許容も確認する必要があります。これによって、列で値の欠落が許容されるか、null 値が必要かが決まります。
テキスト
正しい方法(手動またはデフォルト分割)を決定したら、次のいずれかの方法で Vertex AI にデータを追加できます。
- トレーニング データの準備で指定されているように、パソコンや Cloud Storage から、ラベルを埋め込んだ CSV または JSON Lines 形式のデータをインポートできます。データセットを手動で分割する場合は、CSV または JSON Lines ファイルで分割を指定できます。
- データにまだラベル付けが行われていない場合は、ラベル付けが行われていないテキストのサンプルをアップロードし、Vertex AI コンソールを使用してそれぞれにラベルを適用できます。
動画
データセットに含めたい動画を集めたら、その動画に動画セグメントまたは境界ボックスに関連付けられたラベルが含まれていることを確認する必要があります。動作認識の場合、動画セグメントはタイムスタンプであり、分類の場合、動画セグメントは動画ショット、セグメント、または動画全体のいずれかになります。オブジェクト トラッキングでは、ラベルは境界ボックスに関連付けられます。
動画に境界ボックスとラベルが必要な理由
オブジェクト トラッキングの場合、Vertex AI モデルはパターンを識別することをどのようにして学習するのでしょうか。そのために、トレーニング時に境界ボックスとラベルが必要になります。サッカーの例では、各サンプル動画に検出対象のオブジェクトを囲む境界ボックスが含まれていなければなりません。また、境界ボックスには、該当するアクションに割り当てられた「person」や「ball」などのラベルも必要になります。そのようにしなければ、モデルは何を探せばよいかわからなくなります。サンプル動画にボックスを描画してラベルを割り当てる作業には時間がかかる場合があります。
データにまだラベル付けが行われていない場合は、ラベル付けが行われていない動画をアップロードし、Google Cloud コンソールを使用して境界ボックスとラベルを適用することもできます。詳細については、Google Cloud コンソールを使用してデータにラベルを付けるをご覧ください。
モデルのトレーニング
画像
カスタムモデルの作成時に Vertex AI がどのようにデータセットを使用するかを考慮する
データセットには、トレーニング セット、検証セット、テストセットが含まれます。データセットの分割を指定しない場合(データの準備を参照)、Vertex AI は自動的に画像の 80% をトレーニングに、10% を検証に、10% をテストに使用します。
トレーニング セット
データの大部分をトレーニング セットに含めるようにします。これはトレーニング中にモデルが「見る」データであり、モデルのパラメータ、つまりニューラル ネットワークのノード間の接続の重みを学習するために使用されます。
検証セット
検証セットは「dev」セットとも呼ばれ、やはりトレーニング プロセス中に使用されます。モデルの学習フレームワークは、トレーニング プロセスの各イテレーションでトレーニング データを取り込んだ後、検証セットに対するモデルのパフォーマンスに基づき、そのハイパーパラメータ(モデルの構造を指定する変数)を調整します。トレーニング セットを使用してハイパーパラメータを調整しようとすると、モデルがトレーニング データに過度に適合してしまい、正確に一致しないサンプルを一般化するのが困難になる可能性が高いです。多少違ったデータセットを使ってモデル構造を細かく調整することで、モデルがより適切に一般化されます。
テストセット
テストセットはトレーニング プロセスにはまったく使用されません。モデルのトレーニングが完了したら、モデル向けのまったく新しい課題としてテストセットを使用します。テストセットに対するモデルのパフォーマンスを知ることで、モデルが実世界のデータに対してどのように機能するかをおおよそ理解できます。
手動分割
自分でデータセットを分割することもできます。手動でのデータ分割は、プロセスをより詳細に制御したい場合や、モデルのトレーニング ライフサイクルの特定の部分に特定のサンプルを確実に含めたい場合に適しています。
表形式
データセットをインポートしたら、次のステップはモデルをトレーニングすることです。Vertex AI は、トレーニングのデフォルトを使用して信頼性の高い ML モデルを生成しますが、ユースケースに基づいて一部のパラメータを調整することもできます。
できるだけ多くの特徴列をトレーニングで選択するようにしてください。ただし、それぞれをレビューして、トレーニングに適していることを確認してください。特徴の選択に関しては、以下の事項に留意してください。
- 各行に一意の値を持つランダムに割り当てられた ID 列のように、ノイズを発生させる特徴列を選択しないでください。
- 各特徴列とその値を把握しておいてください。
- 複数のモデルを 1 つのデータセットから作成する場合は、現在の予測問題の一部ではないターゲット列を削除してください。
- 公平性の原則を思い出してください。モデルのトレーニングで使用している特徴は、主流ではないグループにとって偏った、あるいは不公平な意思決定となるでしょうか。
Vertex AI がデータセットを使用する方法
データセットは、トレーニング セット、検証セット、テストセットに分割されます。デフォルトで Vertex AI が適用する分割は、トレーニングするモデルのタイプによって変わります。また、必要に応じて、分割を指定する(手動分割)こともできます。詳細については、AutoML モデルのデータ分割についてをご覧ください。
トレーニング セット
データの大部分をトレーニング セットに含めるようにします。これはトレーニング中にモデルが「見る」データであり、モデルのパラメータ、つまりニューラル ネットワークのノード間の接続の重みを学習するために使用されます。
検証セット
検証セットは「dev」セットとも呼ばれ、やはりトレーニング プロセス中に使用されます。モデルの学習フレームワークは、トレーニング プロセスの各イテレーションでトレーニング データを取り込んだ後、検証セットに対するモデルのパフォーマンスに基づき、そのハイパーパラメータ(モデルの構造を指定する変数)を調整します。トレーニング セットを使用してハイパーパラメータを調整しようとすると、モデルがトレーニング データに過度に適合してしまい、正確に一致しないサンプルを一般化するのが困難になる可能性が高いです。多少違ったデータセットを使ってモデル構造を細かく調整することで、モデルがより適切に一般化されます。
テストセット
テストセットはトレーニング プロセスにはまったく使用されません。モデルのトレーニングが完了したら、Vertex AI はモデル向けのまったく新しい課題としてテストセットを使用します。テストセットに対するモデルのパフォーマンスを知ることで、モデルが実世界のデータに対してどのように機能するかをおおよそ理解できます。
テキスト
カスタムモデルの作成のために Vertex AI がどのようにデータセットを使用するかを考慮する
データセットには、トレーニング セット、検証セット、テストセットが含まれます。分割を指定しない場合(データの準備を参照)、Vertex AI は自動的にコンテンツ ドキュメントの 80% をトレーニングに、10% を検証に、10% をテストに使用します。
トレーニング セット
データの大部分をトレーニング セットに含めるようにします。これはトレーニング中にモデルが「見る」データであり、モデルのパラメータ、つまりニューラル ネットワークのノード間の接続の重みを学習するために使用されます。
検証セット
検証セットは「dev」セットとも呼ばれ、やはりトレーニング プロセス中に使用されます。モデルの学習フレームワークは、トレーニング プロセスの各イテレーションでトレーニング データを取り込んだ後、検証セットに対するモデルのパフォーマンスに基づき、そのハイパーパラメータ(モデルの構造を指定する変数)を調整します。トレーニング セットを使用してハイパーパラメータを調整しようとすると、モデルがトレーニング データに過度に適合してしまい、正確に一致しないサンプルを一般化するのが困難になる可能性が高いです。多少違ったデータセットを使ってモデル構造を細かく調整することで、モデルがより適切に一般化されます。
テストセット
テストセットはトレーニング プロセスにはまったく使用されません。モデルのトレーニングが完了したら、モデル向けのまったく新しい課題としてテストセットを使用します。テストセットに対するモデルのパフォーマンスを知ることで、モデルが実世界のデータに対してどのように機能するかをおおよそ理解できます。
手動分割
自分でデータセットを分割することもできます。手動でのデータ分割は、プロセスをより詳細に制御したい場合や、モデルのトレーニング ライフサイクルの特定の部分に特定のサンプルを確実に含めたい場合に適しています。
動画
トレーニング用の動画データを準備したら、ML モデルの作成に取り掛かることができます。同じデータセットで、異なるモデルの目的に合わせてアノテーション セットを作成できます。アノテーション セットの作成をご覧ください。
Vertex AI の利点の 1 つは、デフォルトのパラメータを使用しても信頼性の高い ML モデルが得られることです。ただし、データの品質と求めている結果に応じてパラメータを調整しなければならない場合もあります。例:
- 予測タイプは、動画を処理する粒度のレベルです。
- フレームレートは、動作認識の場合と同様に、分類対象のラベルが動きの変化の影響を受ける場合に重要です。たとえば、「走っている」と「歩いている」を例として考えてみましょう。フレーム/秒(FPS)が低い場合、「歩いている」クリップが「走っている」ように見える場合があります。オブジェクト トラッキングについても、フレームレートの影響を受けます。基本的には、トラッキングするオブジェクトと、隣接するフレームとの間に十分なオーバーラップが存在する必要があります。
- オブジェクト トラッキングの解像度は、動作認識や動画分類よりも重要です。オブジェクトが小さい場合、必ず高解像度の動画をアップロードしてください。現在のパイプラインでは、通常のトレーニングには 256x256 を使用し、ユーザーデータに小さなオブジェクト(その面積が画像面積の 1% 未満)が多数存在する場合は 512x512 を使用します。256p 以上の動画を使用することをおすすめします。高解像度の動画を使用しても、モデルのパフォーマンスの向上にはつながらない可能性があります。学習や推論の速度を向上させるために、内部的に動画フレームがダウン サンプリングされるためです。
モデルの評価、テスト、デプロイ
モデルを評価する
画像
モデルのトレーニングが完了すると、モデルのパフォーマンスの概要が表示されます。詳細な分析を表示するには、[評価] または [評価全体を見る] をクリックします。
モデルのデバッグは、モデル自体よりもデータを対象とするものです。本番環境への移行前や移行後にパフォーマンスを評価しているとき、モデルが予期しない動作をするようになった場合は、立ち戻ってデータを確認し、改善の余地がある箇所を見つける必要があります。
Vertex AI で実施可能な分析
Vertex AI の評価セクションでは、テストサンプルに対するモデルの出力と一般的な ML の指標を使用してカスタムモデルのパフォーマンスを評価できます。このセクションでは、以下の各コンセプトの意味について説明します。
- モデルの出力
- スコアしきい値
- 真陽性、真陰性、偽陽性、偽陰性
- 適合率と再現率
- 適合率 / 再現率曲線
- 平均適合率
モデルの出力の解釈方法
Vertex AI はテストデータからサンプルを取り出し、モデルに対してまったく新しい課題を提示します。モデルはそれぞれのサンプルについて、各ラベルがそのサンプルにどれくらい強く関連付けられているかを示す一連の数値を出力します。数値が高い場合、高い信頼度でそのラベルをドキュメントに適用できるとモデルが判断したことを示します。
スコアしきい値とは
スコアしきい値を設定することにより、上述の確率をオン / オフの値に変換できます。スコアしきい値とは、モデルで特定のテスト項目にカテゴリを割り当てるために必要な信頼度です。Google Cloud コンソールのスコアしきい値スライダーは、データセット内のすべてのカテゴリと個々のカテゴリのさまざまなしきい値の効果をテストするための視覚的なツールです。スコアしきい値が低い場合、モデルはより多くの画像を分類しますが、いくつかの画像が誤って分類されるリスクがあります。スコアしきい値が低い場合、モデルが分類する画像は少なくなりますが、画像が誤って分類されるリスクが低くなります。Google Cloud コンソールでカテゴリごとにしきい値を微調整してテストできます。ただし、本番環境でモデルを使用するときは、ご自身が最適と判断したしきい値を適用する必要があります。
真陽性、真陰性、偽陽性、偽陰性とは
スコアしきい値を適用すると、モデルの予測は次の 4 つのカテゴリのいずれかになります。
ご自身が最適であると判断したしきい値。
これらのカテゴリを使用して、モデルの有効性の評価に役立つ指標である適合率と再現率を計算できます。
適合率、再現率とは
適合率と再現率は、モデルがどの程度適切に情報を取得しているか、そしてどれだけの情報を除外しているかを把握するのに役立ちます。適合率は、ラベルが割り当てられたすべてのテストサンプルのうち、実際にそのラベルに分類される必要のあるものの数を示します。再現率は、ラベルが割り当てられる必要のあるすべてのテストサンプルのうち、実際にラベルが割り当てられたものの数を示します。
適合率や再現率を高めるために最適化する必要があるか
ユースケースによっては、適合率または再現率を高めるために最適化した方がよい場合もあります。どちらのアプローチが最善かを判断する場合、次の 2 つのユースケースを考慮してください。
ユースケース: 画像のプライバシー
機密情報を自動的に検出し、それをぼかすシステムを作成するとします。
この場合の偽陽性は、ぼかす必要がないものがぼかされることです。目障りですが、弊害はありません。
この場合の偽陰性は、クレジット カードのように、ぼかす必要があるものがぼかされないことです。これは個人情報の盗難につながる恐れがあります。
この場合は、再現率を高めるように最適化することをおすすめします。この指標は、行われたすべての予測において、除外された量を測定します。再現率の高いモデルでは、わずかな関連性を持つサンプルにもラベルを付ける可能性が高いため、カテゴリのトレーニング データが少ない場合に役立ちます。
ユースケース: ストックフォトの検索
特定のキーワードに対応する最適なストックフォトを見つけるシステムを作成するとします。
この場合の偽陽性は、無関係な写真を検出してしまうことです。最も適した画像だけを返すことを謳った検索機能であるため、これは大きな失敗です。
この場合の偽陰性は、キーワード検索に該当する画像を返すのに失敗することです。多くの検索語では、一致する可能性の高い写真が何千枚もあるため、これは問題になりません。
この場合は、適合率を高めるように最適化することをおすすめします。この指標は、行われたすべての予測がどの程度正しいかを測定します。適合率の高いモデルでは、最も関連性の高いサンプルのみにラベルが付けられる可能性が高くなるため、目的のクラスがトレーニング データ内によく見られるケースに有用です。
混同行列の使用
適合率 / 再現率曲線の解釈
スコアしきい値ツールを使用すると、選択したスコアしきい値が適合率と再現率にどう影響するかを調べることができます。スコアしきい値バーのスライダーをドラッグすると、そのしきい値が適合率と再現率のトレードオフ曲線のどこに位置するのか、またそのしきい値が適合率と再現率のそれぞれにどう影響するのかを見ることができます(多分類モデルの場合、これらのグラフの適合率と再現率は、適合率と再現率の指標の計算に使用される唯一のラベルが、返されるラベルのセットの中で最も高いスコアを持つラベルであることを意味します)。これは偽陽性と偽陰性のバランスを取るのに有効です。
モデル全体として許容できると思われるしきい値を選択したら、個々のラベルをクリックし、そのしきい値が各ラベルの適合率 / 再現率曲線のどこに位置するかを確認します。場合によっては、いくつかのラベルで誤った予測が多くなることがあります。その場合は、これらのラベルにカスタマイズした分類別のしきい値を選択できます。たとえば、住宅のデータセットを見た際に、しきい値が 0.5 の場合に、すべての画像タイプに対して合理的な適合率と再現率が得られることに気づいたとします。ただし画像タイプが「Tudor」の場合は例外的にこの傾向が見られませんでした。おそらくこれは「Tudor」が非常に一般的なカテゴリであるためです。このカテゴリでは、偽陽性が数多く見られます。この場合、予測のための分類器を呼び出すときに、「Tudor」のしきい値だけを 0.8 にできます。
平均適合率とは
モデル精度については、適合率 / 再現率曲線の下の領域が有用な指標となります。これは、モデルがすべてのスコアしきい値でどの程度適切に機能するかを測定します。Vertex AI では、この指標を「平均適合率」と呼びます。このスコアが 1.0 に近ければ近いほど、モデルはテストセットに対して適切に機能しています。各ラベルについてランダムに推測するモデルの場合に得られる平均適合率はおよそ 0.5 です。
表形式
モデルのトレーニングが完了すると、モデルのパフォーマンスの概要が表示されます。モデルの評価指標は、データセット(テスト データセット)のスライスに対するモデルのパフォーマンスに基づきます。モデルが実際のデータで使用できる状態になっているかどうかを判断する際には、検討すべき主な指標とコンセプトがあります。
分類指標
スコアしきい値
顧客が来年にジャケットを購入するかどうかを予測する ML モデルを考えてみましょう。特定の顧客がジャケットを購入することを予測する前に、このモデルにはどの程度の確実性が必要でしょうか。分類モデルでは、各予測に信頼スコア(予測クラスが正しいというモデルの確実性の数値的評価)が割り当てられます。スコアしきい値は、特定のスコアがはい / いいえの決定に変換されるときを決定する数値です。つまり、モデルが「はい、この信頼度スコアは、この顧客が来年中にコートを購入すると結論づけるのに十分な高さです」と知らせる値です。
スコアしきい値が低いと、モデルが誤った分類を行うリスクがあります。このため、スコアしきい値は特定のユースケースに基づいて設定しなければなりません。
予測結果
スコアしきい値を適用した後のモデルによる予測は、4 つのカテゴリのうちの 1 つに分類されます。これらのカテゴリを理解するために、もう一度ジャケットのバイナリ分類モデルを考えてみましょう。この例では、陽性のクラス(モデルが予測しようとしていること)は、顧客がジャケットを来年に購入するということです。
- 真陽性: モデルは陽性のクラスを正しく予測している。モデルは顧客がジャケットを購入することを正しく予測しました。
- 偽陽性: モデルは陽性のクラスを誤って予測している。モデルは顧客がジャケットを購入すると予測しましたが、顧客は購入しませんでした。
- 真陰性: モデルは陰性のクラスを正しく予測している。モデルは顧客がジャケットを購入しないことを正しく予測しました。
- 偽陰性: モデルは陰性のクラスを誤って予測している。モデルは顧客がジャケットを購入しないと予測しましたが、顧客は購入しました。
適合率と再現率
適合率と再現率の指標は、モデルがどの程度適切に情報を取得しているか、そしてどれだけの情報を除外しているかを把握するのに役立ちます。適合率と再現率の詳細。
- 適合率は、肯定の予測のうち、正しかった予測の割合です。つまり、顧客が購入するであろうと予測されたうち、実際に購入した割合です。
- 再現率は、モデルによって正しく予測された、このラベルが付けられた行の割合です。つまり、識別できるはずの顧客の購入のうち、実際に識別された割合を示します。
ユースケースに応じて、適合率または再現率の最適化が必要になる場合があります。
その他の分類指標
- AUC PR: 適合率 / 再現率(PR)曲線の下の面積。この値は範囲が 0~1 で、値が高いほど高品質のモデルであることを示します。
- AUC ROC: 受信者操作特性(ROC)曲線の下の面積。この範囲は 0~1 で、値が高いほど高品質のモデルであることを示します。
- 精度: 正しいモデルによって生成された分類予測の割合。
- ログ損失: モデル予測とターゲット値の間のクロス エントロピー。この範囲はゼロから無限大までで、値が小さいほど高品質のモデルであることを示します。
- F1 スコア: 適合率と再現率の調和平均。適合率と再現率のバランスを求めていて、クラス分布が不均一な場合、F1 は有用な指標となります。
予測と回帰の指標
モデルを構築すると、Vertex AI から提供されるさまざまな標準指標を使ってそのモデルを評価できます。モデルを評価する方法について完全な答えはありません。問題の種類や、モデルで達成したいことを合わせて評価指標を検討します。Vertex AI で利用できる指標の一部の概要を次に示します。
平均絶対誤差(MAE)
MAE は、ターゲットと予測値の平均絶対差です。一連の予測における誤差(ターゲットと予測値の差)の平均の大きさを測定します。MAE では、絶対値を使用するため、関係の方向性を考慮せず、過少パフォーマンスや過剰パフォーマンスが示されることもありません。MAE を評価するとき、値が小さいほど品質の高いモデルを示します(0 は完全な予測因子を表します)。
二乗平均平方根誤差(RMSE)
RMSE は、ターゲットと予測値の平均二乗差の平方根です。RMSE は MAE よりも外れ値の影響を受けやすいため、大きな誤差が心配な場合は RMSE のほうがより有用な評価指標といえます。MAE と同様、値が小さいほど品質の高いモデルを示します(0 は完全な予測因子を表します)。
二乗平均対数平方誤差(RMSLE)
RMSLE は対数目盛りの RMSE です。RMSLE は絶対誤差よりも相対誤差に対して敏感であり、過剰パフォーマンスよりも過小パフォーマンスを重視します。
観測された分位値(予測のみ)
特定のターゲット分位値の場合、観測された分位値では、指定された分位予測値の下に観測された値の実際の割合が示されます。観測された分位値は、モデルがターゲット分位値とどれくらい離れているかを示します。2 つの値の差が小さいほど、高品質なモデルであることを示します。
スケールされたピンボールロス(予測のみ)
指定されたターゲット分位値でのモデルの品質を測定します。数値が小さいほど、高品質のモデルであることを示します。さまざまな分位値のスケールされたピンボールロス指標を比較することで、そうした分位値間のモデルの相対精度を判断できます。
テキスト
モデルのトレーニングが完了すると、モデルのパフォーマンスの概要が表示されます。詳細な分析を表示するには、[評価] または [評価全体を見る] をクリックします。
モデルを評価する前の留意点
モデルのデバッグは、モデル自体よりもデータを対象とするものです。本番環境への移行前や移行後にモデルのパフォーマンスを評価しているときに、モデルが予期しない動作をするようになった場合は、いったん戻ってデータをチェックし、改善が必要な箇所を見つける必要があります。
Vertex AI で実施可能な分析
Vertex AI の評価セクションでは、テストサンプルに対するモデルの出力と一般的な ML の指標を使用してカスタムモデルのパフォーマンスを評価できます。このセクションでは、次の各コンセプトについて説明します。
- モデルの出力
- スコアしきい値
- 真陽性、真陰性、偽陽性、偽陰性
- 適合率と再現率
- 適合率 / 再現率曲線
- 平均適合率
モデルの出力の解釈方法
Vertex AI はテストデータからサンプルを取り出し、モデルに対して新しい課題を提示します。モデルはそれぞれのサンプルについて、各ラベルがそのサンプルにどれくらい強く関連付けられているかを示す一連の数値を出力します。数値が高い場合、高い信頼度でそのラベルをドキュメントに適用できるとモデルが判断したことを示します。
スコアしきい値とは
スコアしきい値を使用して、Vertex AI で確率をオン / オフの値に変換できます。スコアしきい値とは、モデルで特定のテスト項目にカテゴリを割り当てるために必要な信頼度です。コンソールのスコアしきい値スライダーは、データセット内のさまざまなしきい値の影響をテストするための視覚的なツールです。上述の例では、すべてのカテゴリのスコアしきい値を 0.8 に設定した場合、「Great Service」と「Suggestion」は割り当てられますが、「Info Request」は割り当てられません。スコアしきい値が低い場合、モデルはより多くのテキスト項目を分類しますが、より多くのテキスト項目が誤って分類されるリスクがあります。スコアしきい値が高い場合、モデルが分類するテキスト項目は少なくなりますが、誤って分類されるリスクも低くなります。Google Cloud コンソールでカテゴリごとにしきい値を微調整してテストできます。ただし、本番環境でモデルを使用するときは、ご自身が最適と判断したしきい値を適用する必要があります。
真陽性、真陰性、偽陽性、偽陰性とは
スコアしきい値を適用すると、モデルの予測は次の 4 つのカテゴリのいずれかになります。
これらのカテゴリを使用して、モデルの有効性の評価に役立つ指標である適合率と再現率を計算できます。
適合率、再現率とは
適合率と再現率は、モデルがどの程度適切に情報を取得しているか、そしてどれだけの情報を除外しているかを把握するのに役立ちます。適合率は、ラベルが割り当てられたすべてのテストサンプルのうち、実際にそのラベルに分類される必要のあるものの数を示します。再現率は、ラベルが割り当てられる必要のあるすべてのテストサンプルのうち、実際にラベルが割り当てられたものの数を示します。
適合率や再現率を高めるために最適化する必要があるか
ユースケースによっては、適合率または再現率を高めるために最適化した方がよい場合もあります。どちらのアプローチが最善かを判断する場合、次の 2 つのユースケースを考慮してください。
ユースケース: 緊急のドキュメント
たとえば、緊急を要するドキュメントを他よりも優先するシステムを作成するとします。
この場合の偽陽性とは、緊急ではないドキュメントが緊急を要するものとして分類されることです。ユーザーはこれを緊急でないとして却下し、先に進むことができます。
この場合の偽陰性とは、緊急を要するドキュメントがそのように分類されないことです。これは問題を引き起こす可能性があります。
この場合は、再現率を高めるように最適化することをおすすめします。この指標は、行われたすべての予測において、除外された量を測定します。再現率の高いモデルでは、わずかな関連性を持つサンプルにもラベルを付ける可能性が高いため、カテゴリのトレーニング データが少ない場合に役立ちます。
ユースケース: スパムフィルタ
たとえば、スパムメールとそうでないメッセージを自動でフィルタするシステムを作成するとします。
この場合の偽陰性とは、スパムメールが検出されず、受信トレイに表示されることです。通常、これによってユーザーが受ける影響は、少しの不快感といった程度です。
この場合の偽陽性とは、メールが誤ってスパムとして分類され、受信トレイから削除されることです。それが重要なメールだった場合、ユーザーに重大な影響が及ぶ危険性があります。
この場合は、適合率を高めるように最適化することをおすすめします。この指標は、行われたすべての予測がどの程度正しいかを測定します。適合率の高いモデルは、最も関連性の高いサンプルのみにラベルを付ける可能性が高いため、目的のカテゴリがトレーニング データ内によく見られる場合に便利です。
混同行列の使用
混同行列を使用すると、各ラベルに対するモデルのパフォーマンスを比較できます。理想的なモデルでは、対角線上のすべての値が高くなり、他の値はすべて低くなります。これは、目的のカテゴリが正しく識別されていることを示しています。他の値が高い場合は、モデルがテスト項目をどう誤って分類しているかを知るための手がかりとなります。
適合率 / 再現率曲線の解釈
スコアしきい値ツールを使用すると、選択したスコアしきい値が適合率と再現率にどう影響するかを調べることができます。スコアしきい値バーのスライダーをドラッグすると、そのしきい値が適合率と再現率のトレードオフ曲線のどこに位置するのか、またそのしきい値が適合率と再現率のそれぞれにどう影響するのかを見ることができます(多分類モデルの場合、これらのグラフの適合率と再現率は、適合率と再現率の指標の計算に使用される唯一のラベルが、返されるラベルのセットの中で最も高いスコアを持つラベルであることを意味します)。これは、偽陽性と偽陰性のバランスを取るのに役立ちます。
モデル全体で許容できると思われるしきい値を選択したら、個々のラベルをクリックし、そのしきい値が各ラベルの適合率 / 再現率曲線のどこに位置するかを確認できます。場合によっては、いくつかのラベルで誤った予測が多くなることがあります。その場合は、これらのラベルにカスタマイズした分類別のしきい値を選択できます。たとえば、顧客のコメントのデータセットを見た際に、しきい値が 0.5 の場合に、すべてのコメントタイプに対して合理的な適合率と再現率が得られることに気付いたとします。ただしコメントタイプが「Suggestion」の場合は例外的にこの傾向が見られませんでした。おそらくこれは「Suggestion」が非常に一般的なカテゴリであるためです。このカテゴリでは、偽陽性が数多く見られます。この場合、予測のための分類子を呼び出すときに、「Suggestion」のしきい値だけを 0.8 にできます。
平均適合率とは
モデル精度については、適合率 / 再現率曲線の下の領域が有用な指標となります。これは、モデルがすべてのスコアしきい値でどの程度適切に機能するかを測定します。Vertex AI では、この指標を「平均適合率」と呼びます。このスコアが 1.0 に近ければ近いほど、モデルはテストセットに対して適切に機能しています。各ラベルについてランダムに推測するモデルの場合に得られる平均適合率はおよそ 0.5 です。
動画
モデルのトレーニングが完了すると、モデルのパフォーマンスの概要が表示されます。モデルの評価指標は、データセット(テスト データセット)のスライスに対するモデルのパフォーマンスに基づきます。モデルが新しいデータで使用できる状態になっているかどうかを判断する際に検討すべき重要な指標とコンセプトがいくつかあります。
スコアしきい値
ML モデルは、特定の動画の種類がゴールであることをどのようにして判断しているのでしょうか。各予測には、信頼スコアが割り当てられます。信頼スコアは、モデルの確実性を表す数値評価であり、所定の動画セグメントに特定のクラスが含まれている確度を示します。スコアしきい値は、所定のスコアが「はい / いいえ」という肯否に結び付けられるポイント(数値)です。つまり、「信頼スコアの数値がこれだけ高ければ、この動画セグメントはゴールシーンである」とモデルで結論付けることができます。
スコアしきい値が低いと、モデルが動画セグメントに誤ったラベルを付けるリスクがあります。このため、スコアしきい値は特定のユースケースに基づいて設定しなければなりません。医療の癌検出においてラベルを貼り違えるのと、スポーツ動画でのミスでは重大性は大きく異なります。癌検出などのミスが許されない用途では、スコアしきい値を高く設定してください。
予測結果
スコアしきい値を適用した後のモデルによる予測は、4 つのカテゴリのうちの 1 つに分類されます。これらのカテゴリを理解するために、特定のセグメントがサッカーのゴールシーンであるかどうかを検出するモデルについて考えてみましょう。この例では、ゴールは陽性のクラス(モデルが予測しようとしているクラス)です。
- 真陽性: モデルは陽性のクラスを正しく予測している。モデルは動画セグメントがゴールシーンであると正しく予測しました。
- 偽陽性: モデルは陽性のクラスを誤って予測している。モデルはセグメントがゴールシーンであると予測しましたが、実際にはゴールシーンではありませんでした。
- 真陰性: モデルは陰性のクラスを正しく予測している。モデルはセグメントがゴールシーンでないと正しく予測しました。
- 偽陰性: モデルは陰性のクラスを誤って予測している。モデルはセグメントがゴールシーンでないと予測しましたが、実際にはゴールシーンでした。
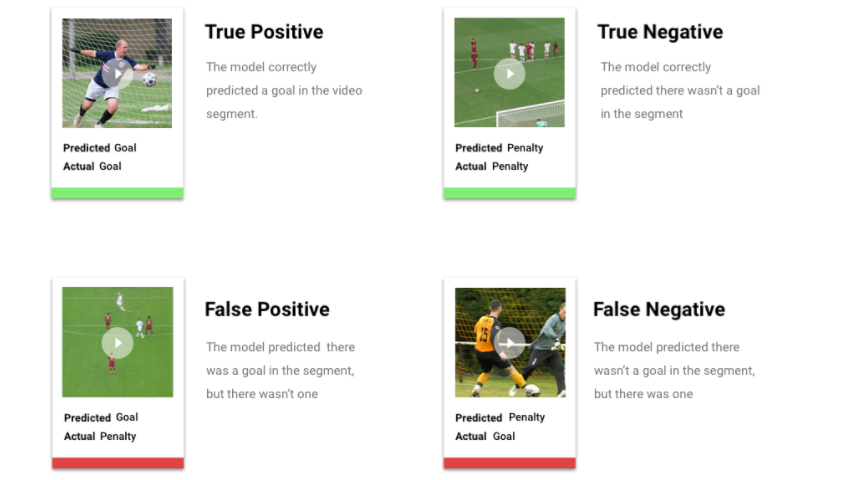
適合率と再現率
適合率と再現率の指標は、モデルがどの程度適切に情報を取得しているか、そしてどれだけの情報を除外しているかを把握するのに役立ちます。適合率と再現率の詳細。
- 適合率は、肯定の予測のうち、正しかった予測の割合です。つまり、「ゴール」のラベルが付けられたすべての予測のうち、実際にゴールシーンが含まれていた予測の割合を示します。
- 再現率は、すべての肯定の予測のうち、実際に識別された予測の割合です。つまり、識別できるはずのゴールシーンのうち、実際に識別された割合を示します。
ユースケースに応じて、適合率または再現率の最適化が必要になる場合があります。以下のようなユースケースが考えられます。
ユースケース: 動画に含まれる個人情報
たとえば、動画に含まれる機密情報を自動的に検出し、その情報をぼかすソフトウェアを作成しているとします。誤った結果には、次の影響が伴います。
- この場合の偽陽性は、ぼかす必要がない情報が識別され、ぼかされてしまうことです。目障りですが、弊害はありません。
- この場合の偽陰性は、クレジット カード番号のような、ぼかさなければならない情報の識別に失敗することです。これは個人情報の漏洩につながるため、最悪のシナリオです。
このユースケースでは、モデルが関連するケースをすべて検出できるよう、再現率を高くする必要があります。再現率の高いモデルでは、関連性の低いサンプルだけでなく、誤ったサンプルにもラベルが付けられる可能性(ぼかす必要がない情報もぼかされる可能性)が高くなります。
ユースケース: ストック動画の検索
ユーザーがキーワードで動画ライブラリを検索できるソフトウェアを作成するとします。次の誤った結果について考えてみましょう。
- この場合の偽陽性は、関連性のない動画が返されることです。このシステムでは関連する動画だけを結果として返すことを目的としているため、ソフトウェアはその目的を果たしていないことになります。
- この場合の偽陰性は、関連する動画が返されないことです。多くのキーワードは何百本もの動画に関連するため、関連性のない動画が返されるほど問題ではありません。
この例では、モデルが関連性のある正しい結果を出すよう、適合率を高くする必要があります。適合率の高いモデルでは、最も関連性の高いサンプルにのみラベルが付けられる可能性が高くなりますが、一部の適切なサンプルも除外されることがあります。モデルの評価指標の詳細
モデルのテスト
画像
Vertex AI は、自動的にデータの 10%(ただしデータ分割を自分で行った場合は、選択したパーセント)を使ってモデルをテストします。テストデータに対するモデルのパフォーマンスは [評価] ページに表示されます。モデルの信頼度をチェックしたい場合は、いくつかの方法があります。最も簡単な方法は、[デプロイとテスト] ページにいくつかの画像をアップロードし、サンプルに対してモデルが選択するラベルを確認することです。モデルの性能が良ければ、これで期待に沿った結果が得られるはずです。受け取ると予想される各種画像のサンプルをいくつか試してみてください。
モデルを独自の自動テストで使用する場合、[デプロイとテスト] ページでは、モデルをプログラムで呼び出す方法も示されています。
表形式
モデル指標の評価とは主として、モデルをデプロイする準備ができているかどうかを判断する方法ですが、新しいデータでテストすることもできます。新しいデータをアップロードして、モデルの予測が自分の予想と一致するかどうかを確認してください。評価指標や新しいデータによるテストに基づいて、モデルのパフォーマンスを引き続き向上させる必要があることがあります。
テキスト
Vertex AI は、自動的にデータの 10%(ただしデータ分割を自分で行った場合は、選択したパーセント)を使ってモデルをテストします。テストデータに対するモデルのパフォーマンスは [評価] ページに表示されます。モデルをチェックしたい場合は、いくつかの方法があります。モデルをデプロイしたら、[デプロイとテスト] ページのフィールドにテキスト サンプルを入力し、サンプルに対してモデルが選択するラベルを確認します。モデルの性能が良ければ、これで期待に沿った結果が得られるはずです。受け取ると予想される各種コメントのサンプルをいくつか試してみてください。
モデルを独自の自動テストで使用する場合、[デプロイとテスト] ページに、モデルをプログラムから呼び出す方法を示すサンプル API リクエストが示されます。
モデルを独自の自動テストで使用する場合、[デプロイとテスト] ページに、モデルをプログラムから呼び出す方法を示すサンプル API リクエストが示されます。
[バッチ予測] ページでバッチ予測を作成し、多数の予測リクエストを 1 つにまとめることができます。バッチ予測は非同期です。つまり、モデルはすべての予測リクエストを処理するまで待機してから結果を返します。
動画
Vertex AI は、データの 20%(データ分割を自分で行った場合は、選択したパーセンテージ)を自動的に使用して、モデルのテストを行います。コンソールの [評価] タブには、テストデータに対するモデルのパフォーマンスが表示されます。また、モデルのチェックを行う場合のために、サニティ チェックの方法もいくつか用意されています。その 1 つが、[テストと使用] タブでテスト用の動画データを含む CSV ファイルを提供し、それらの動画に対してモデルが予測したラベルを確認する方法です。モデルの性能が良ければ、これで期待に沿った結果が得られるはずです。
さらに、予測の可視化のしきい値を調整できるほか、予測結果を 1 秒間隔、ショット境界自動検出後のビデオカメラ ショット、動画セグメント全体といった 3 つの時間スケールで調べることもできます。
モデルのデプロイ
画像
モデルのパフォーマンスが満足のいくものになったら、モデルの使用を開始します。本番環境で使用することになることも、一回限りの予測リクエストになることもあります。ユースケースに応じて、モデルをさまざまな方法で使用できます。
バッチ予測
バッチ予測は、多数の予測リクエストを一度で行うのに便利です。バッチ予測は非同期です。つまり、モデルはすべての予測リクエストを処理するのを待ってから、予測値を含む JSON Lines ファイルを返します。
オンライン予測
モデルをデプロイして、REST API を使用して予測リクエストで使用できるようにします。オンライン予測は同期的(リアルタイム)です。つまり、予測はすぐに返されますが、API 呼び出しごとに 1 つの予測リクエストしか受け付けません。オンライン予測は、モデルがアプリケーションの一部であり、システムの一部が素早い予測ターンアラウンドに依存している場合に便利です。
表形式
モデルのパフォーマンスが満足のいくものになったら、モデルの使用を開始します。本番環境で使用することになることも、一回限りの予測リクエストになることもあります。ユースケースに応じて、モデルをさまざまな方法で使用できます。
バッチ予測
バッチ予測は、多数の予測リクエストを一度で行うのに便利です。バッチ予測は非同期です。つまり、モデルはすべての予測リクエストを処理するのを待ってから、予測値を含む CSV ファイルまたは BigQuery テーブルを返します。
オンライン予測
モデルをデプロイして、REST API を使用して予測リクエストで使用できるようにします。オンライン予測は同期的(リアルタイム)です。つまり、予測はすぐに返されますが、API 呼び出しごとに 1 つの予測リクエストしか受け付けません。オンライン予測は、モデルがアプリケーションの一部であり、システムの一部が素早い予測ターンアラウンドに依存している場合に便利です。
動画
モデルのパフォーマンスが満足のいくものになったら、モデルの使用を開始します。Vertex AI はバッチ予測を使用します。バッチ予測を使用すると、Cloud Storage でホストされている動画へのパスを含む CSV ファイルをアップロードできます。モデルは各動画を処理し、別の CSV ファイルに予測を出力します。バッチ予測は非同期オペレーションです。つまり、モデルはすべての予測リクエストを処理してから、結果を出力します。
クリーンアップ
不要な料金が発生しないようにするため、モデルを使用していないときはモデルをデプロイ解除してください。
モデルの使用が終了したら、アカウントに不要な料金が発生しないように、作成したリソースを削除します。
- Hello 画像データ: プロジェクトのクリーンアップ
- Hello 表形式データ: プロジェクトのクリーンアップ
- Hello テキストデータ: プロジェクトのクリーンアップ
- Hello 動画データ: プロジェクトのクリーンアップ