If you set an API key requirement in your API, requests to the protected method, class, or API are rejected unless they have a key generated in your project or within other projects belonging to developers with whom you have granted access to enable your API. The project that the API key was created in is not logged and is not added to the request header. You can, however, view the Google Cloud project that a client is associated with on the Endpoints Services page, as described in Filter for a specific consumer project.
For information on which Google Cloud project an API key should be created in, see Sharing APIs protected by API key.
Restricting access to all API methods
To require an API key for all calls into the API, add
apiKeyRequired = AnnotationBoolean.TRUE
to your
@Api annotation.
For example:
@Api(
name = "echo",
version = "v1",
apiKeyRequired = AnnotationBoolean.TRUE
)
public class Echo {
//API class and methods...
}
Restricting access to specific API methods
To require an API key for all calls into a specific API method, add
apiKeyRequired = AnnotationBoolean.TRUE
to your
@ApiMethod annotation.
For example:
To require an API key for all calls into a specific API class, add
apiKeyRequired = AnnotationBoolean.TRUE
to your @ApiClass
annotation.
Removing API key restriction for a method
To turn off API key validation for an API or API method, remove
api_key_required=True
(Python) or apiKeyRequired = AnnotationBoolean.TRUE
(Java) from your API or method decorator or annotation. Then recompile and
re-deploy.
Calling an API using an API key
If an API or API method requires an API key, supply the key using a query
parameter named key
, as shown in this cURL example:
curl \
-H "Content-Type: application/json" \
-X POST \
-d '{"message": "echo"}' \
"${HOST}/_ah/api/echo/v1/echo_api_key?key=${API_KEY}"
where HOST
and API_KEY
are variables containing your API host
name and API key, respectively. Replace echo
with the name of your API, and
v1
with the version of your API.
Sharing APIs protected by API key
API keys are associated with the Google Cloud project in which they have been created. If you have decided to require an API key for your API, the Google Cloud project that the API key gets created in depends on the answers to the following questions:
- Do you need to distinguish between the callers of your API so that you can use Endpoints features such as quotas?
- Do all the callers of your API have their own Google Cloud projects?
- Do you need to set up different API key restrictions?
You can use the following decision tree as a guide for deciding which Google Cloud project to create the API key in.
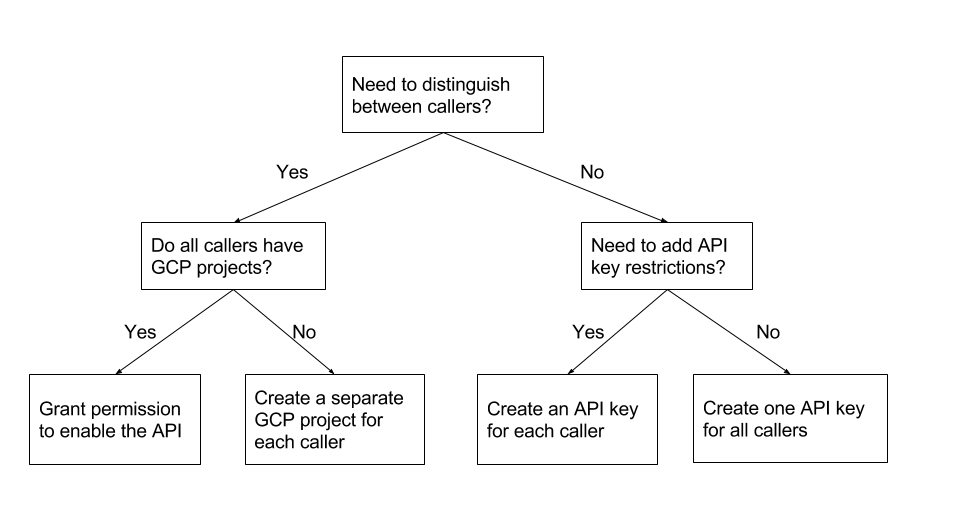
Grant permission to enable the API
When you need to distinguish between callers of your API, and each caller has their own Google Cloud project, you can grant principals permission to enable the API in their own Google Cloud project. This way, users of your API can create their own API key for use with your API.
For example, suppose your team has created an API for internal use by various client programs in your company, and each client program has their own Google Cloud project. To distinguish between callers of your API, the API key for each caller must be created in a different Google Cloud project. You can grant your coworkers permission to enable the API in the Google Cloud project that the client program is associated with.
To let users create their own API key:
- In the Google Cloud project in which your API is configured, grant each user the permission to enable your API.
- Contact the users, and let them know that they can enable your API in their own Google Cloud project and create an API key.
Create a separate Google Cloud project for each caller
When you need to distinguish between callers of your API, and not all of the callers have Google Cloud projects, you can create a separate Google Cloud project and API key for each caller. Before creating the projects, give some thought to the project names so that you can easily identify the caller associated with the project.
For example, suppose you have external customers of your API, and you have no idea how the client programs that call your API were created. Perhaps some of the clients use Google Cloud services and have a Google Cloud project, and perhaps some don't. To distinguish between the callers, you must create a separate Google Cloud project and API key for each caller.
To create a separate Google Cloud project and API key for each caller:
- Create a separate project for each caller.
- In each project, enable your API and create an API key.
- Give the API key to each caller.
Create an API key for each caller
When you don't need to distinguish between callers of your API, but you want to add API key restrictions, you can create a separate API key for each caller in the same project.
To create an API key for each caller in the same project:
- In either the project that your API is configured in, or a project that your API is enabled in, create an API key for each customer that has the API key restrictions that you need.
- Give the API key to each caller.
Create one API key for all callers
When you don't need to distinguish between callers of your API, and you don't need to add API restrictions, but you still want to require an API key (to prevent anonymous access, for example), you can create one API key for all callers to use.
To create one API key for all callers:- In either the project that your API is configured in, or a project that your API is enabled in, create an API key for all caller.
- Give the same API key to every caller.