ロゴ検出は、よく知られている商品のロゴを画像から検出します。
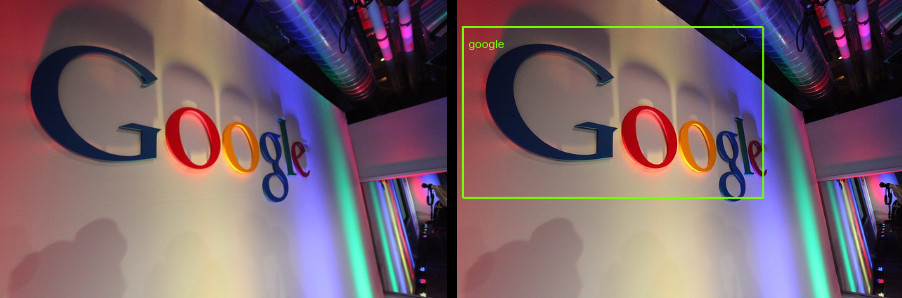
ロゴ検出リクエスト
Google Cloud プロジェクトと認証のセットアップ
ローカル画像でのロゴの検出
Vision API を使用して、ローカル画像ファイルに特徴検出を実行できます。
REST リクエストの場合は、リクエストの本文で画像ファイルのコンテンツを base64 エンコード文字列として送信します。
gcloud
とクライアント ライブラリ リクエストの場合は、リクエストにローカル イメージへのパスを指定します。
REST
リクエストのデータを使用する前に、次のように置き換えます。
- BASE64_ENCODED_IMAGE: バイナリ画像データの base64 表現(ASCII 文字列)。これは次のような文字列になります。
/9j/4QAYRXhpZgAA...9tAVx/zDQDlGxn//2Q==
- PROJECT_ID: Google Cloud プロジェクト ID
HTTP メソッドと URL:
POST https://vision.googleapis.com/v1/images:annotate
リクエストの本文(JSON):
{ "requests": [ { "image": { "content": "BASE64_ENCODED_IMAGE" }, "features": [ { "type": "LOGO_DETECTION" }, ] } ] }
リクエストを送信するには、次のいずれかのオプションを選択します。
curl
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
リクエストが成功すると、サーバーは 200 OK
HTTP ステータス コードと JSON 形式のレスポンスを返します。
対処:
{ "responses": [ { "logoAnnotations": [ { "mid": "/m/045c7b", "description": "google", "score": 0.980325, "boundingPoly": { "vertices": [ { "x": 12, "y": 42 }, { "x": 439, "y": 42 }, { "x": 439, "y": 285 }, { "x": 12, "y": 285 } ] } } ] } ] }
Go
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Go の設定手順を完了してください。詳細については、Vision Go API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Java
このサンプルを試す前に、Vision API クイックスタート: クライアント ライブラリの使用の Java の設定手順を完了してください。詳細については、Vision API Java のリファレンス ドキュメントをご覧ください。
Node.js
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Node.js の設定手順を完了してください。 詳細については、Vision Node.js API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Python
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Python の設定手順を完了してください。 詳細については、Vision Python API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
その他の言語
C#: クライアント ライブラリ ページの C# の設定手順を行ってから、.NET 用の Vision リファレンス ドキュメントをご覧ください。
PHP: クライアント ライブラリ ページの PHP の設定手順を行ってから、PHP 用の Vision リファレンス ドキュメントをご覧ください。
Ruby: クライアント ライブラリ ページの Ruby の設定手順を行ってから、Ruby 用の Vision リファレンス ドキュメントをご覧ください。
リモート画像でのロゴの検出
Vision API を使用すると、Cloud Storage またはウェブ上にあるリモート画像ファイルに特徴検出を実行できます。リモート ファイル リクエストを送信するには、リクエストの本文でファイルのウェブ URL または Cloud Storage URI を指定します。
REST
リクエストのデータを使用する前に、次のように置き換えます。
- CLOUD_STORAGE_IMAGE_URI: Cloud Storage バケット内の有効な画像ファイルへのパス。少なくとも、ファイルに対する読み取り権限が必要です。例:
gs://cloud-samples-data/vision/logo/google_logo.jpg
- PROJECT_ID: Google Cloud プロジェクト ID。
HTTP メソッドと URL:
POST https://vision.googleapis.com/v1/images:annotate
リクエストの本文(JSON):
{ "requests": [ { "image": { "source": { "gcsImageUri": "CLOUD_STORAGE_IMAGE_URI" } }, "features": [ { "type": "LOGO_DETECTION" }, ] } ] }
リクエストを送信するには、次のいずれかのオプションを選択します。
curl
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
リクエストが成功すると、サーバーは 200 OK
HTTP ステータス コードと JSON 形式のレスポンスを返します。
対処:
{ "responses": [ { "logoAnnotations": [ { "mid": "/m/045c7b", "description": "google", "score": 0.980325, "boundingPoly": { "vertices": [ { "x": 12, "y": 42 }, { "x": 439, "y": 42 }, { "x": 439, "y": 285 }, { "x": 12, "y": 285 } ] } } ] } ] }
Go
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Go の設定手順を完了してください。詳細については、Vision Go API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Java
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Java の設定手順を完了してください。 詳細については、Vision Java API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Node.js
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Node.js の設定手順を完了してください。 詳細については、Vision Node.js API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Python
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Python の設定手順を完了してください。 詳細については、Vision Python API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
gcloud
画像プロパティの検出を行うには、次の例で示すように gcloud ml vision detect-logos
コマンドを使用します。
gcloud ml vision detect-logos gs://cloud-samples-data/vision/logo/google_logo.jpg
その他の言語
C#: クライアント ライブラリ ページの C# の設定手順を行ってから、.NET 用の Vision リファレンス ドキュメントをご覧ください。
PHP: クライアント ライブラリ ページの PHP の設定手順を行ってから、PHP 用の Vision リファレンス ドキュメントをご覧ください。
Ruby: クライアント ライブラリ ページの Ruby の設定手順を行ってから、Ruby 用の Vision リファレンス ドキュメントをご覧ください。
試してみる
下の画像でロゴの検出をお試しください。前のセクションで指定した画像(gs://cloud-samples-data/vision/logo/google_logo.jpg
)を使用しても構いませんし、ご自分の画像を指定して試してみるのもよいでしょう。[実行] を選択してリクエストを送信します。
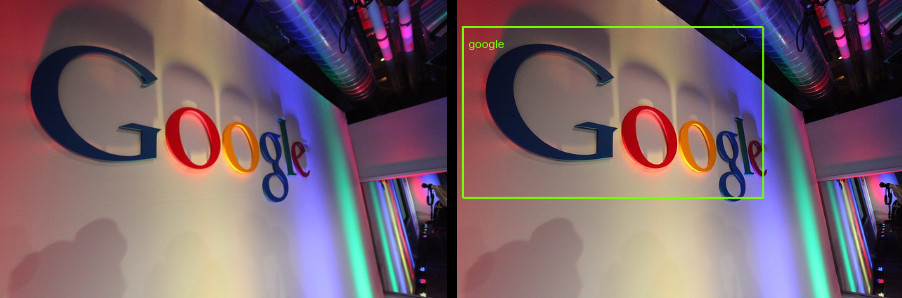
リクエストの本文:
{ "requests": [ { "features": [ { "type": "LOGO_DETECTION" } ], "image": { "source": { "imageUri": "gs://cloud-samples-data/vision/logo/google_logo.jpg" } } } ] }