受众
本教程旨在帮助您开发具有 Vision API Web 检测功能的应用。本教程假定您熟悉基本的编程结构和技术,不过,即使您是初级程序员,也应该能够毫不费力地根据本教程进行操作,然后按照 Vision API 参考文档的说明创建基本应用。
本教程将逐步介绍一个 Vision API 应用,展示如何通过调用 Vision API 来使用其 Web 检测功能。
前提条件
- 在 Google Cloud Console 中设置 Vision API 项目。
设置您的环境以便使用应用默认凭据。
Python
- 安装 Python。
- 安装 pip。
- 安装 Google Cloud 客户端库。
概览
本教程介绍了一个使用 Web detection
请求的基本 Vision API 应用。Web detection
响应使用以下各项对请求中发送的图片进行注释:
- 从 Web 获取的标签
- 具有匹配图片的网站网址
- 与请求中的图片部分匹配或完全匹配的网络图片的网址
- 视觉上相似的图片的网址
代码清单
我们建议您在阅读代码时参照 Vision API Python 参考文档以便理解。
此简单应用执行以下任务:
- 导入运行应用所需的库
- 将图片路径作为参数传递给
main()
函数 - 使用 Google Cloud API 客户端执行 Web 检测
- 循环执行响应并输出结果
- 输出具有说明和分数的网络实体的列表
- 输出匹配页面的列表
- 输出部分匹配的图片的列表
- 输出完全匹配的图片的列表
深入了解
导入库
导入标准库:
argparse
:允许应用接受输入文件名作为参数io
:从文件读取内容
其他导入:
google.cloud.vision
库中的ImageAnnotatorClient
类,用于访问 Vision API。google.cloud.vision
库中的types
模块,用于构建请求。
运行应用
这里,我们仅解析用于指定 Web 图片网址的传入参数,并将其传递给 main()
函数。
向 API 进行身份验证
您必须先使用先前获得的凭据向 Vision API 服务进行身份验证,然后才能与该服务通信。在应用中,获取凭据的最简单方法是使用应用默认凭据 (ADC)。客户端库会自动获取凭据。默认情况下,此操作通过从 GOOGLE_APPLICATION_CREDENTIALS
环境变量获取凭据来完成,该变量应设置为指向您的服务账号的 JSON 密钥文件(如需了解详情,请参阅设置服务账号)。
构建请求
现在,Vision API 服务已准备就绪,我们可以构建要发送至该服务的请求。
此代码段执行以下任务:
- 创建
ImageAnnotatorClient
实例作为客户端。 - 通过本地文件或 URI 构建
Image
对象。 - 将
Image
对象传递给客户端的web_detection
方法。 - 返回注释。
输出响应
操作完成后,我们可以浏览 WebDetection,并输出注释中包含的实体和网址(每种注释类型的前两条结果将在下一部分展示)。
运行应用
要运行该应用,我们将传入以下汽车图片的网址 (http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg)。
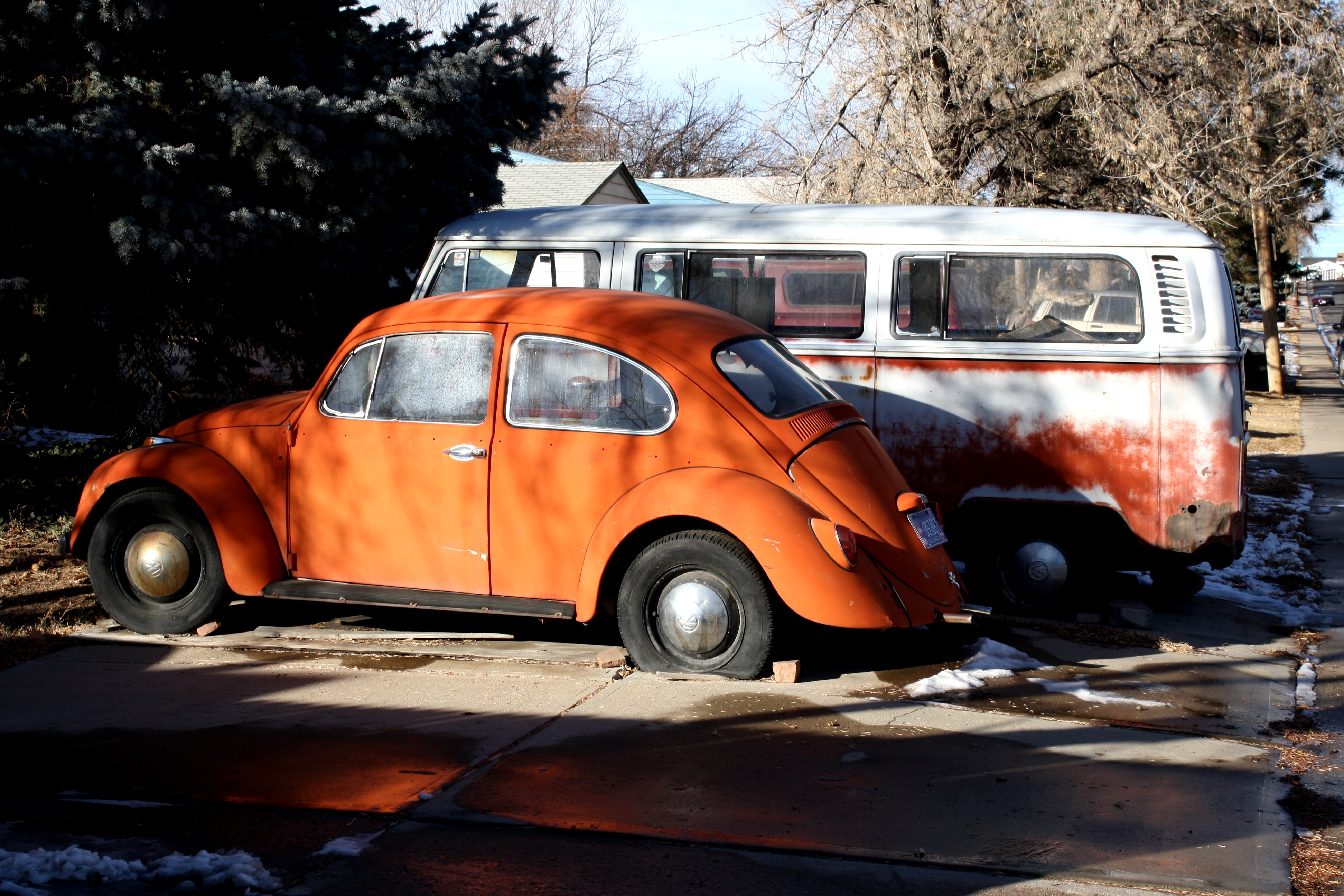
以下是包含所传入汽车图片网址的 Python 命令,后跟控制台输出。请注意,所列实体后添加了相关性分数。另请注意,这些分数未标准化,在不同图片查询之间也不具有可比性。
python web_detect.py "http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg"
5 Pages with matching images retrieved Url : http://www.photos-public-domain.com/2011/01/07/old-volkswagen-bug-and-van/ Url : http://pix-hd.com/old+volkswagen+van+for+sale ... 2 Full Matches found: Url : http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg Url : http://www.wbwagen.com/media/old-volkswagen-bug-and-van-picture-free-photograph-photos-public_s_66f487042adad5a6.jpg 4 Partial Matches found: Url : http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg Url : http://www.wbwagen.com/media/old-vw-bug-and-vanjpg_s_ac343d7f041b5f8d.jpg ... 5 Web entities found: Score : 5.35028934479 Description: Volkswagen Beetle Score : 1.43998003006 Description: Volkswagen Score : 0.828279972076 Description: Volkswagen Type 2 Score : 0.75271999836 Description: Van Score : 0.690039992332 Description: Car
恭喜!您已使用 Vision API 执行 Web 检测!