地标检测功能可检测图片内热门的自然景观和人造建筑。
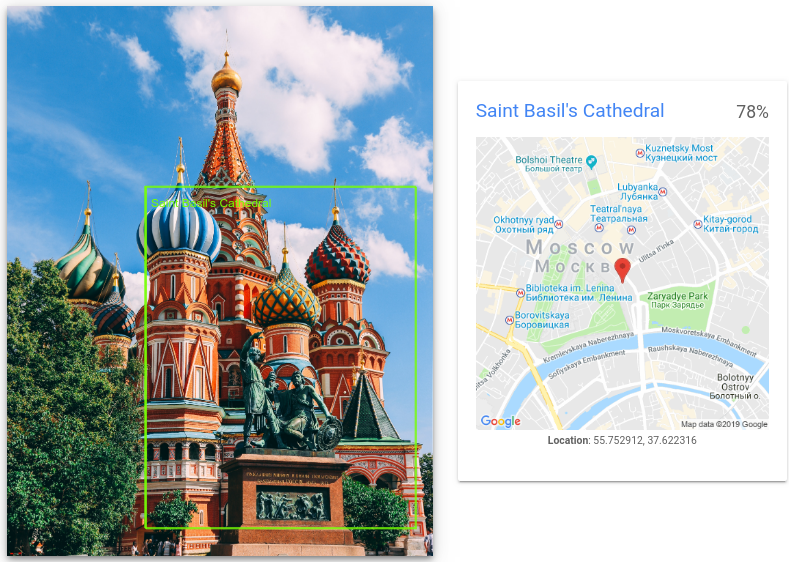
地标检测请求
设置您的 Google Cloud 项目和身份验证
检测本地图片中的地标
您可以使用 Vision API 对本地图片文件执行特征检测。
对于 REST 请求,请将图片文件的内容作为 base64 编码的字符串在请求正文中发送。
对于 gcloud
和客户端库请求,请在请求中指定本地图片的路径。
REST
在使用任何请求数据之前,请先进行以下替换:
- BASE64_ENCODED_IMAGE:二进制图片数据的 base64 表示(ASCII 字符串)。此字符串应类似于以下字符串:
/9j/4QAYRXhpZgAA...9tAVx/zDQDlGxn//2Q==
- RESULTS_INT:(可选)要返回的结果的整数值。如果您省略
"maxResults"
字段及其值,则 API 会默认返回 10 个结果。此字段不适用于以下功能类型:TEXT_DETECTION
、DOCUMENT_TEXT_DETECTION
或CROP_HINTS
。 - PROJECT_ID:您的 Google Cloud 项目 ID。
HTTP 方法和网址:
POST https://vision.googleapis.com/v1/images:annotate
请求 JSON 正文:
{ "requests": [ { "image": { "content": "BASE64_ENCODED_IMAGE" }, "features": [ { "maxResults": RESULTS_INT, "type": "LANDMARK_DETECTION" }, ] } ] }
如需发送请求,请选择以下方式之一:
curl
将请求正文保存在名为 request.json
的文件中,然后执行以下命令:
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
将请求正文保存在名为 request.json
的文件中,然后执行以下命令:
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
如果请求成功,服务器将返回一个 200 OK
HTTP 状态代码以及 JSON 格式的响应。
响应:
{ "responses": [ { "landmarkAnnotations": [ { "mid": "/m/014lft", "description": "Saint Basil's Cathedral", "score": 0.7840959, "boundingPoly": { "vertices": [ { "x": 812, "y": 1058 }, { "x": 2389, "y": 1058 }, { "x": 2389, "y": 3052 }, { "x": 812, "y": 3052 } ] }, "locations": [ { "latLng": { "latitude": 55.752912, "longitude": 37.622315883636475 } } ] } ] } ] }
Go
试用此示例之前,请按照《Vision 快速入门:使用客户端库》中的 Go 设置说明进行操作。 如需了解详情,请参阅 Vision Go API 参考文档。
如需向 Vision 进行身份验证,请设置应用默认凭据。 如需了解详情,请参阅为本地开发环境设置身份验证。
Java
在试用此示例之前,请按照Vision API 快速入门:使用客户端库中的 Java 设置说明进行操作。如需了解详情,请参阅 Vision API Java 参考文档。
Node.js
试用此示例之前,请按照《Vision 快速入门:使用客户端库》中的 Node.js 设置说明进行操作。 如需了解详情,请参阅 Vision Node.js API 参考文档。
如需向 Vision 进行身份验证,请设置应用默认凭据。 如需了解详情,请参阅为本地开发环境设置身份验证。
Python
试用此示例之前,请按照《Vision 快速入门:使用客户端库》中的 Python 设置说明进行操作。 如需了解详情,请参阅 Vision Python API 参考文档。
如需向 Vision 进行身份验证,请设置应用默认凭据。 如需了解详情,请参阅为本地开发环境设置身份验证。
其他语言
C#: 请按照客户端库页面上的 C# 设置说明操作,然后访问 .NET 版 Vision 参考文档。
PHP: 请按照客户端库页面上的 PHP 设置说明操作,然后访问 PHP 版 Vision 参考文档。
Ruby 版: 请按照客户端库页面上的 Ruby 设置说明操作,然后访问 Ruby 版 Vision 参考文档。
检测远程图片中的地标
您可以使用 Vision API 对位于 Cloud Storage 或网络中的远程图片文件执行特征检测。如需发送远程文件请求,请在请求正文中指定文件的网址或 Cloud Storage URI。
REST
在使用任何请求数据之前,请先进行以下替换:
- CLOUD_STORAGE_IMAGE_URI:Cloud Storage 存储桶中有效图片文件的路径。您必须至少拥有该文件的读取权限。
示例:
gs://cloud-samples-data/vision/landmark/st_basils.jpeg
- RESULTS_INT:(可选)要返回的结果的整数值。如果您省略
"maxResults"
字段及其值,则 API 会默认返回 10 个结果。此字段不适用于以下功能类型:TEXT_DETECTION
、DOCUMENT_TEXT_DETECTION
或CROP_HINTS
。 - PROJECT_ID:您的 Google Cloud 项目 ID。
HTTP 方法和网址:
POST https://vision.googleapis.com/v1/images:annotate
请求 JSON 正文:
{ "requests": [ { "image": { "source": { "gcsImageUri": "CLOUD_STORAGE_IMAGE_URI" } }, "features": [ { "maxResults": RESULTS_INT, "type": "LANDMARK_DETECTION" }, ] } ] }
如需发送请求,请选择以下方式之一:
curl
将请求正文保存在名为 request.json
的文件中,然后执行以下命令:
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
将请求正文保存在名为 request.json
的文件中,然后执行以下命令:
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
如果请求成功,服务器将返回一个 200 OK
HTTP 状态代码以及 JSON 格式的响应。
响应:
{ "responses": [ { "landmarkAnnotations": [ { "mid": "/m/014lft", "description": "Saint Basil's Cathedral", "score": 0.7840959, "boundingPoly": { "vertices": [ { "x": 812, "y": 1058 }, { "x": 2389, "y": 1058 }, { "x": 2389, "y": 3052 }, { "x": 812, "y": 3052 } ] }, "locations": [ { "latLng": { "latitude": 55.752912, "longitude": 37.622315883636475 } } ] } ] } ] }
Go
试用此示例之前,请按照《Vision 快速入门:使用客户端库》中的 Go 设置说明进行操作。 如需了解详情,请参阅 Vision Go API 参考文档。
如需向 Vision 进行身份验证,请设置应用默认凭据。 如需了解详情,请参阅为本地开发环境设置身份验证。
Java
试用此示例之前,请按照《Vision 快速入门:使用客户端库》中的 Java 设置说明进行操作。 如需了解详情,请参阅 Vision Java API 参考文档。
如需向 Vision 进行身份验证,请设置应用默认凭据。 如需了解详情,请参阅为本地开发环境设置身份验证。
Node.js
试用此示例之前,请按照《Vision 快速入门:使用客户端库》中的 Node.js 设置说明进行操作。 如需了解详情,请参阅 Vision Node.js API 参考文档。
如需向 Vision 进行身份验证,请设置应用默认凭据。 如需了解详情,请参阅为本地开发环境设置身份验证。
Python
试用此示例之前,请按照《Vision 快速入门:使用客户端库》中的 Python 设置说明进行操作。 如需了解详情,请参阅 Vision Python API 参考文档。
如需向 Vision 进行身份验证,请设置应用默认凭据。 如需了解详情,请参阅为本地开发环境设置身份验证。
gcloud
如需执行地标检测,请使用 gcloud ml vision detect-landmarks
命令,如以下示例所示:
gcloud ml vision detect-landmarks gs://cloud-samples-data/vision/landmark/st_basils.jpeg
其他语言
C#: 请按照客户端库页面上的 C# 设置说明操作,然后访问 .NET 版 Vision 参考文档。
PHP: 请按照客户端库页面上的 PHP 设置说明操作,然后访问 PHP 版 Vision 参考文档。
Ruby 版: 请按照客户端库页面上的 Ruby 设置说明操作,然后访问 Ruby 版 Vision 参考文档。
试用
接下来,请尝试执行地标检测。您可以使用已指定的图片 (gs://cloud-samples-data/vision/landmark/st_basils.jpeg
) 或指定您自己的图片。选择执行即可发送请求。
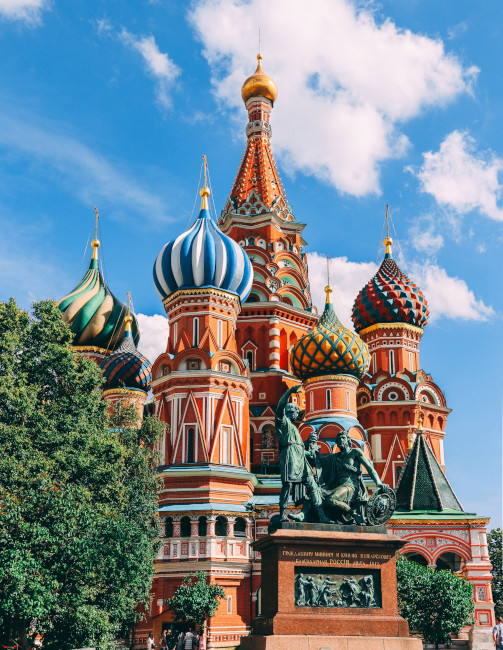
请求正文:
{ "requests": [ { "features": [ { "maxResults": 10, "type": "LANDMARK_DETECTION" } ], "image": { "source": { "imageUri": "gs://cloud-samples-data/vision/landmark/st_basils.jpeg" } } } ] }