Vision API では、オブジェクト ローカライズを使用して、画像内の複数のオブジェクトを検出して抽出できます。
オブジェクト ローカライズにより、画像内のオブジェクトが識別され、オブジェクトごとに LocalizedObjectAnnotation が指定されます。LocalizedObjectAnnotation
ごとに、オブジェクトに関する情報、オブジェクトの位置、画像内でオブジェクトがある領域の四角い境界線が識別されます。
オブジェクト ローカライズでは、画像内で目立っているオブジェクトとそれほど目立たないオブジェクトの両方が識別されます。
オブジェクト情報は英語でのみ返されます。Cloud Translation は、英語のラベルを他の言語に翻訳できます。
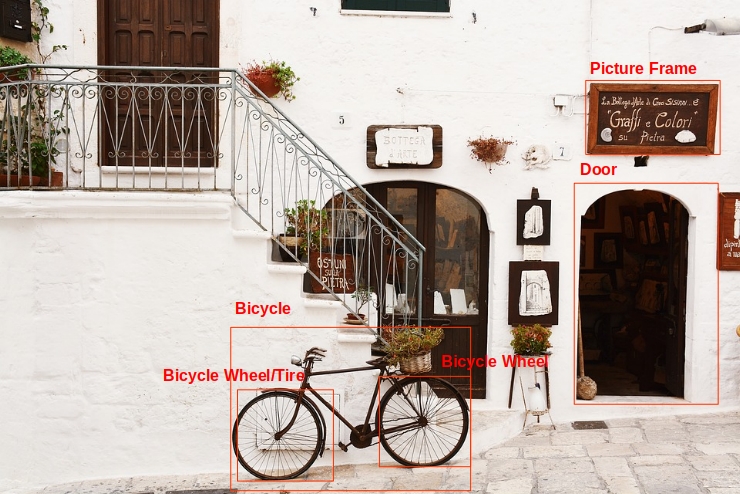
たとえば、API は前の画像のオブジェクトについて、次の情報と境界の位置情報を返します。
名前 | mid | スコア | 境界 |
---|---|---|---|
Bicycle wheel | /m/01bqk0 | 0.89648587 | (0.32076266, 0.78941387), (0.43812272, 0.78941387), (0.43812272, 0.97331065), (0.32076266, 0.97331065) |
Bicycle | /m/0199g | 0.886761 | (0.312, 0.6616471), (0.638353, 0.6616471), (0.638353, 0.9705882), (0.312, 0.9705882) |
Bicycle wheel | /m/01bqk0 | 0.6345275 | (0.5125398, 0.760708), (0.6256646, 0.760708), (0.6256646, 0.94601655), (0.5125398, 0.94601655) |
Picture frame | /m/06z37_ | 0.6207608 | (0.79177403, 0.16160682), (0.97047985, 0.16160682), (0.97047985, 0.31348917), (0.79177403, 0.31348917) |
Tire | /m/0h9mv | 0.55886006 | (0.32076266, 0.78941387), (0.43812272, 0.78941387), (0.43812272, 0.97331065), (0.32076266, 0.97331065) |
Door | /m/02dgv | 0.5160098 | (0.77569866, 0.37104446), (0.9412425, 0.37104446), (0.9412425, 0.81507325), (0.77569866, 0.81507325) |
mid には、ラベルの Google Knowledge Graph エントリに対応する MID(Machine-generated Identifier)が格納されます。mid の値の調べ方については、Google Knowledge Graph Search API のドキュメントをご覧ください。
使ってみる
Google Cloud を初めて使用される方は、アカウントを作成して、実際のシナリオでの Cloud Vision API のパフォーマンスを評価してください。新規のお客様には、ワークロードの実行、テスト、デプロイができる無料クレジット $300 分を差し上げます。
Cloud Vision API の無料トライアルオブジェクト ローカライズのリクエスト
Google Cloud プロジェクトと認証のセットアップ
ローカル画像でのオブジェクトの検出
Vision API を使用して、ローカル画像ファイルに特徴検出を実行できます。
REST リクエストの場合は、リクエストの本文で画像ファイルのコンテンツを base64 エンコード文字列として送信します。
gcloud
とクライアント ライブラリ リクエストの場合は、リクエストにローカル イメージへのパスを指定します。
REST
リクエストのデータを使用する前に、次のように置き換えます。
- BASE64_ENCODED_IMAGE: バイナリ画像データの base64 表現(ASCII 文字列)。これは次のような文字列になります。
/9j/4QAYRXhpZgAA...9tAVx/zDQDlGxn//2Q==
- RESULTS_INT: (省略可)返される結果の整数値。
"maxResults"
フィールドとその値を省略した場合、API はデフォルト値の 10 を返します。このフィールドは、TEXT_DETECTION
、DOCUMENT_TEXT_DETECTION
、CROP_HINTS
の各機能タイプには適用されません。 - PROJECT_ID: Google Cloud プロジェクト ID。
HTTP メソッドと URL:
POST https://vision.googleapis.com/v1/images:annotate
リクエストの本文(JSON):
{ "requests": [ { "image": { "content": "BASE64_ENCODED_IMAGE" }, "features": [ { "maxResults": RESULTS_INT, "type": "OBJECT_LOCALIZATION" }, ] } ] }
リクエストを送信するには、次のいずれかのオプションを選択します。
curl
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
リクエストが成功すると、サーバーは 200 OK
HTTP ステータス コードと JSON 形式のレスポンスを返します。
レスポンス:
Go
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Go の設定手順を完了してください。 詳細については、Vision Go API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Java
このサンプルを試す前に、Vision API クイックスタート: クライアント ライブラリの使用の Java の設定手順を完了してください。詳細については、Vision API Java のリファレンス ドキュメントをご覧ください。
Node.js
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Node.js の設定手順を完了してください。 詳細については、Vision Node.js API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Python
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Python の設定手順を完了してください。 詳細については、Vision Python API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
その他の言語
C#: クライアント ライブラリ ページの C# の設定手順を行ってから、.NET 用の Vision リファレンス ドキュメントをご覧ください。
PHP: クライアント ライブラリ ページの PHP の設定手順を行ってから、PHP 用の Vision リファレンス ドキュメントをご覧ください。
Ruby: クライアント ライブラリ ページの Ruby の設定手順を行ってから、Ruby 用の Vision リファレンス ドキュメントをご覧ください。
リモート画像内のオブジェクトの検出
Vision API を使用すると、Cloud Storage またはウェブ上にあるリモート画像ファイルに特徴検出を実行できます。リモート ファイル リクエストを送信するには、リクエストの本文でファイルのウェブ URL または Cloud Storage URI を指定します。
REST
リクエストのデータを使用する前に、次のように置き換えます。
- CLOUD_STORAGE_IMAGE_URI: Cloud Storage バケット内の有効な画像ファイルへのパス。少なくとも、ファイルに対する読み取り権限が必要です。例:
https://cloud.google.com/vision/docs/images/bicycle_example.png
- RESULTS_INT: (省略可)返される結果の整数値。
"maxResults"
フィールドとその値を省略した場合、API はデフォルト値の 10 を返します。このフィールドは、TEXT_DETECTION
、DOCUMENT_TEXT_DETECTION
、CROP_HINTS
の各機能タイプには適用されません。 - PROJECT_ID: Google Cloud プロジェクト ID。
HTTP メソッドと URL:
POST https://vision.googleapis.com/v1/images:annotate
リクエストの本文(JSON):
{ "requests": [ { "image": { "source": { "imageUri": "CLOUD_STORAGE_IMAGE_URI" } }, "features": [ { "maxResults": RESULTS_INT, "type": "OBJECT_LOCALIZATION" }, ] } ] }
リクエストを送信するには、次のいずれかのオプションを選択します。
curl
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
リクエストが成功すると、サーバーは 200 OK
HTTP ステータス コードと JSON 形式のレスポンスを返します。
レスポンス:
Go
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Go の設定手順を完了してください。 詳細については、Vision Go API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Java
このサンプルを試す前に、Vision API クイックスタート: クライアント ライブラリの使用の Java の設定手順を完了してください。詳細については、Vision API Java のリファレンス ドキュメントをご覧ください。
Node.js
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Node.js の設定手順を完了してください。 詳細については、Vision Node.js API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Python
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Python の設定手順を完了してください。 詳細については、Vision Python API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
gcloud
画像内のラベルを検出するには、次の例で示すように gcloud ml vision detect-objects
コマンドを実行します。
gcloud ml vision detect-objects https://cloud.google.com/vision/docs/images/bicycle_example.png
その他の言語
C#: クライアント ライブラリ ページの C# の設定手順を行ってから、.NET 用の Vision リファレンス ドキュメントをご覧ください。
PHP: クライアント ライブラリ ページの PHP の設定手順を行ってから、PHP 用の Vision リファレンス ドキュメントをご覧ください。
Ruby: クライアント ライブラリ ページの Ruby の設定手順を行ってから、Ruby 用の Vision リファレンス ドキュメントをご覧ください。
試してみる
次のツールを使用して、オブジェクト検出とローカライズをお試しください。前のセクションで指定した画像(https://cloud.google.com/vision/docs/images/bicycle_example.png
)を使用しても構いませんし、ご自分の画像を指定して試してみるのもよいでしょう。[実行] を選択してリクエストを送信します。
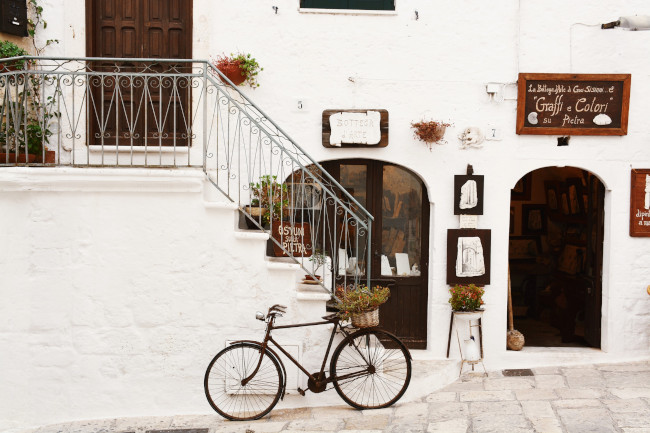
リクエストの本文:
{ "requests": [ { "features": [ { "maxResults": 10, "type": "OBJECT_LOCALIZATION" } ], "image": { "source": { "imageUri": "https://cloud.google.com/vision/docs/images/bicycle_example.png" } } } ] }