Vision API では、画像内のエンティティについての情報を、幅広いカテゴリにわたって検出、抽出できます。
ラベルにより、一般的な物体、場所、活動、動物の種類、商品などを識別できます。ターゲットを設定したカスタムラベルが必要な場合、Cloud AutoML Vision では、カスタム機械学習モデルをトレーニングして画像を分類できます。
ラベルは英語でのみ返されます。Cloud Translate API は、英語のラベルを多数の他言語のいずれかに翻訳できます。
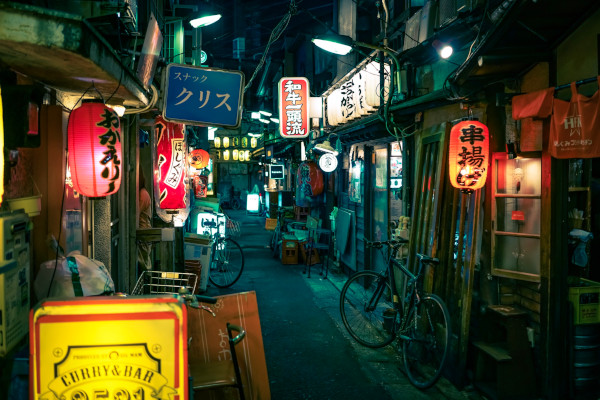
たとえば、上の画像の場合は、次のラベルのリストが返されます。
説明 | スコア |
---|---|
街路 | 0.872 |
スナップショット | 0.852 |
町 | 0.848 |
夜 | 0.804 |
路地 | 0.713 |
ラベル検出のリクエスト
Google Cloud プロジェクトと認証のセットアップ
ローカル画像でラベルを検出する
Vision API を使用して、ローカル画像ファイルに特徴検出を実行できます。
REST リクエストの場合は、リクエストの本文で画像ファイルのコンテンツを base64 エンコード文字列として送信します。
gcloud
とクライアント ライブラリ リクエストの場合は、リクエストにローカル イメージへのパスを指定します。
REST
リクエストのデータを使用する前に、次のように置き換えます。
- BASE64_ENCODED_IMAGE: バイナリ画像データの base64 表現(ASCII 文字列)。これは次のような文字列になります。
/9j/4QAYRXhpZgAA...9tAVx/zDQDlGxn//2Q==
- RESULTS_INT: (省略可)返される結果の整数値。
"maxResults"
フィールドとその値を省略した場合、API はデフォルト値の 10 を返します。このフィールドは、TEXT_DETECTION
、DOCUMENT_TEXT_DETECTION
、CROP_HINTS
の各機能タイプには適用されません。 - PROJECT_ID: Google Cloud プロジェクト ID。
HTTP メソッドと URL:
POST https://vision.googleapis.com/v1/images:annotate
リクエストの本文(JSON):
{ "requests": [ { "image": { "content": "BASE64_ENCODED_IMAGE" }, "features": [ { "maxResults": RESULTS_INT, "type": "LABEL_DETECTION" } ] } ] }
リクエストを送信するには、次のいずれかのオプションを選択します。
curl
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
リクエストが成功すると、サーバーは 200 OK
HTTP ステータス コードと JSON 形式のレスポンスを返します。
LABEL_DETECTION
レスポンスには、検出されたラベル、スコア、トピカリティ、不透明ラベル ID が含まれます。
mid
: 存在する場合は、このエンティティの Google Knowledge Graph エントリに対応する MID(Machine-generated Identifier)が格納されます。mid
の値は異なる言語間でも一意であるため、異なる言語のエンティティをまとめるために使用することもできます。MID 値を調べるには、Google Knowledge Graph API のドキュメントをご覧ください。description
- ラベルの説明。score
- 信頼スコア。0(信頼できない)から 1(信頼度が非常に高い)の範囲で示されます。topicality
- 画像に対する ICA(Image Content Annotation)ラベルの関連度。ページの全体的なコンテキストに対するラベルの重要度 / 関連度を測定します。
{ "responses": [ { "labelAnnotations": [ { "mid": "/m/01c8br", "description": "Street", "score": 0.87294734, "topicality": 0.87294734 }, { "mid": "/m/06pg22", "description": "Snapshot", "score": 0.8523099, "topicality": 0.8523099 }, { "mid": "/m/0dx1j", "description": "Town", "score": 0.8481104, "topicality": 0.8481104 }, { "mid": "/m/01d74z", "description": "Night", "score": 0.80408716, "topicality": 0.80408716 }, { "mid": "/m/01lwf0", "description": "Alley", "score": 0.7133322, "topicality": 0.7133322 } ] } ] }
Go
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Go の設定手順を完了してください。 詳細については、Vision Go API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Java
このサンプルを試す前に、Vision API クイックスタート: クライアント ライブラリの使用の Java の設定手順を完了してください。詳細については、Vision API Java のリファレンス ドキュメントをご覧ください。
Node.js
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Node.js の設定手順を完了してください。 詳細については、Vision Node.js API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Python
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Python の設定手順を完了してください。 詳細については、Vision Python API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
その他の言語
C#: クライアント ライブラリ ページの C# の設定手順を行ってから、.NET 用の Vision リファレンス ドキュメントをご覧ください。
PHP: クライアント ライブラリ ページの PHP の設定手順を行ってから、PHP 用の Vision リファレンス ドキュメントをご覧ください。
Ruby: クライアント ライブラリ ページの Ruby の設定手順を行ってから、Ruby 用の Vision リファレンス ドキュメントをご覧ください。
リモート画像でラベルを検出する
Vision API を使用すると、Cloud Storage またはウェブ上にあるリモート画像ファイルに特徴検出を実行できます。リモート ファイル リクエストを送信するには、リクエストの本文でファイルのウェブ URL または Cloud Storage URI を指定します。
REST
リクエストのデータを使用する前に、次のように置き換えます。
- CLOUD_STORAGE_IMAGE_URI: Cloud Storage バケット内の有効な画像ファイルへのパス。少なくとも、ファイルに対する読み取り権限が必要です。例:
gs://cloud-samples-data/vision/label/setagaya.jpeg
- RESULTS_INT: (省略可)返される結果の整数値。
"maxResults"
フィールドとその値を省略した場合、API はデフォルト値の 10 を返します。このフィールドは、TEXT_DETECTION
、DOCUMENT_TEXT_DETECTION
、CROP_HINTS
の各機能タイプには適用されません。 - PROJECT_ID: Google Cloud プロジェクト ID。
HTTP メソッドと URL:
POST https://vision.googleapis.com/v1/images:annotate
リクエストの本文(JSON):
{ "requests": [ { "image": { "source": { "gcsImageUri": "CLOUD_STORAGE_IMAGE_URI" } }, "features": [ { "maxResults": RESULTS_INT, "type": "LABEL_DETECTION" }, ] } ] }
リクエストを送信するには、次のいずれかのオプションを選択します。
curl
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
curl -X POST \
-H "Authorization: Bearer $(gcloud auth print-access-token)" \
-H "x-goog-user-project: PROJECT_ID" \
-H "Content-Type: application/json; charset=utf-8" \
-d @request.json \
"https://vision.googleapis.com/v1/images:annotate"
PowerShell
リクエスト本文を request.json
という名前のファイルに保存して、次のコマンドを実行します。
$cred = gcloud auth print-access-token
$headers = @{ "Authorization" = "Bearer $cred"; "x-goog-user-project" = "PROJECT_ID" }
Invoke-WebRequest `
-Method POST `
-Headers $headers `
-ContentType: "application/json; charset=utf-8" `
-InFile request.json `
-Uri "https://vision.googleapis.com/v1/images:annotate" | Select-Object -Expand Content
リクエストが成功すると、サーバーは 200 OK
HTTP ステータス コードと JSON 形式のレスポンスを返します。
LABEL_DETECTION
レスポンスには、検出されたラベル、スコア、トピカリティ、不透明ラベル ID が含まれます。
mid
: 存在する場合は、このエンティティの Google Knowledge Graph エントリに対応する MID(Machine-generated Identifier)が格納されます。mid
の値は異なる言語間でも一意であるため、異なる言語のエンティティをまとめるために使用することもできます。MID 値を調べるには、Google Knowledge Graph API のドキュメントをご覧ください。description
- ラベルの説明。score
- 信頼スコア。0(信頼できない)から 1(信頼度が非常に高い)の範囲で示されます。topicality
- 画像に対する ICA(Image Content Annotation)ラベルの関連度。ページの全体的なコンテキストに対するラベルの重要度 / 関連度を測定します。
{ "responses": [ { "labelAnnotations": [ { "mid": "/m/01c8br", "description": "Street", "score": 0.87294734, "topicality": 0.87294734 }, { "mid": "/m/06pg22", "description": "Snapshot", "score": 0.8523099, "topicality": 0.8523099 }, { "mid": "/m/0dx1j", "description": "Town", "score": 0.8481104, "topicality": 0.8481104 }, { "mid": "/m/01d74z", "description": "Night", "score": 0.80408716, "topicality": 0.80408716 }, { "mid": "/m/01lwf0", "description": "Alley", "score": 0.7133322, "topicality": 0.7133322 } ] } ] }
Go
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Go の設定手順を完了してください。 詳細については、Vision Go API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Java
このサンプルを試す前に、Vision API クイックスタート: クライアント ライブラリの使用の Java の設定手順を完了してください。詳細については、Vision API Java のリファレンス ドキュメントをご覧ください。
Node.js
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Node.js の設定手順を完了してください。 詳細については、Vision Node.js API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
Python
このサンプルを試す前に、Vision クイックスタート: クライアント ライブラリの使用にある Python の設定手順を完了してください。 詳細については、Vision Python API のリファレンス ドキュメントをご覧ください。
Vision に対する認証を行うには、アプリケーションのデフォルト認証情報を設定します。詳細については、ローカル開発環境の認証を設定するをご覧ください。
gcloud
画像内のラベルを検出するには、次の例で示すように gcloud ml vision detect-labels
コマンドを実行します。
gcloud ml vision detect-labels gs://cloud-samples-data/vision/label/setagaya.jpeg
その他の言語
C#: クライアント ライブラリ ページの C# の設定手順を行ってから、.NET 用の Vision リファレンス ドキュメントをご覧ください。
PHP: クライアント ライブラリ ページの PHP の設定手順を行ってから、PHP 用の Vision リファレンス ドキュメントをご覧ください。
Ruby: クライアント ライブラリ ページの Ruby の設定手順を行ってから、Ruby 用の Vision リファレンス ドキュメントをご覧ください。
試してみる
ラベルの検出を試してみましょう。すでに指定済みの画像(gs://cloud-samples-data/vision/label/setagaya.jpeg
)を使用することも、独自の画像を指定することもできます。[実行] を選択してリクエストを送信します。
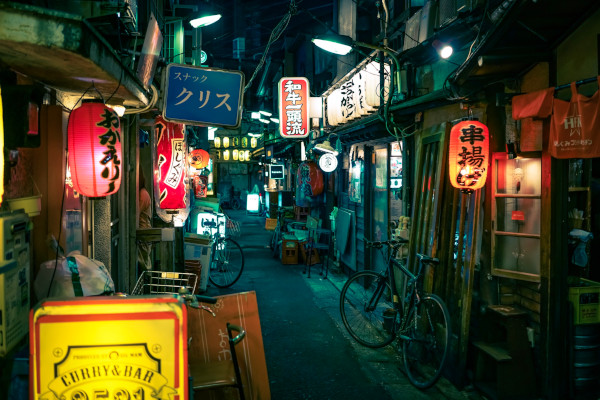
リクエストの本文:
{ "requests": [ { "features": [ { "maxResults": 5, "type": "LABEL_DETECTION" } ], "image": { "source": { "imageUri": "gs://cloud-samples-data/vision/label/setagaya.jpeg" } } } ] }