대상
이 가이드의 목표는 사용자가 Vision API 웹 감지 기능을 사용하는 애플리케이션을 개발하도록 돕는 것입니다. 프로그램의 구조와 기법에 대한 기초적인 지식을 보유한 사용자를 대상으로 하지만, 초보 프로그래머라도 어려움 없이 가이드를 따라 실행해 보고, Vision API 참조 문서를 활용하여 기본적인 애플리케이션을 만들 수 있도록 구성되어 있습니다.
이 가이드에서는 Vision API 애플리케이션을 단계별로 설명하면서 Vision API를 호출하여 웹 감지 기능을 사용하는 방법을 보여줍니다.
기본 요건
- Google Cloud Console에서 Vision API 프로젝트를 설정합니다.
애플리케이션 기본 사용자 인증 정보를 사용할 환경을 설정합니다.
Python
- Python을 설치합니다.
- pip를 설치합니다.
- Google Cloud 클라이언트 라이브러리를 설치합니다.
개요
이 가이드에서는 Web detection
요청을 사용하는 기본 Vision API 애플리케이션에 대해 설명합니다. Web detection
응답은 요청에 포함하여 전송된 이미지에 다음을 주석으로 추가합니다.
- 웹에서 가져온 라벨
- 일치 이미지가 있는 사이트 URL
- 요청의 이미지와 부분적으로 일치하거나 완전히 일치하는 웹 이미지의 URL
- 시각적으로 유사한 이미지의 URL
코드 목록
코드를 보다가 잘 이해되지 않는 부분은 Vision API Python 참조에서 확인하시기 바랍니다.
이 간단한 애플리케이션은 다음과 같은 작업을 수행합니다.
- 애플리케이션을 실행하는 데 필요한 라이브러리 가져오기
- 이미지 경로를 인수로 사용하여
main()
함수에 전달 - Google Cloud API 클라이언트를 사용하여 웹 감지 수행
- 응답을 루프로 반복하면서 결과 출력
- 웹 항목의 목록, 설명, 점수 출력
- 일치 페이지 목록 출력
- 부분 일치 이미지 목록 출력
- 완전 일치 이미지 목록 출력
자세히 살펴보기
라이브러리 가져오기
표준 라이브러리 가져오기:
argparse
: 애플리케이션에서 입력 파일 이름을 인수로 사용io
: 파일에서 읽기
기타 가져오기:
google.cloud.vision
라이브러리의ImageAnnotatorClient
클래스: Vision API에 액세스google.cloud.vision
라이브러리의types
모듈: 요청 생성
애플리케이션 실행
여기에서는 웹 이미지의 URL을 지정하는 전달된 인수를 간단히 파싱하여 main()
함수에 전달합니다.
API 인증
Vision API 서비스와 통신하려면 우선 이전에 획득한 사용자 인증 정보를 사용하여 서비스를 인증해야 합니다. 애플리케이션 내에서 사용자 인증 정보를 얻는 가장 간단한 방법은 애플리케이션 기본 사용자 인증 정보(ADC)를 사용하는 것입니다. 클라이언트 라이브러리는 사용자 인증 정보를 자동으로 가져옵니다. 이를 위해 기본적으로 GOOGLE_APPLICATION_CREDENTIALS
환경 변수에서 사용자 인증 정보를 가져오며, 이 환경 변수는 서비스 계정의 JSON 키 파일을 가리키도록 설정해야 합니다. 자세한 내용은 서비스 계정 설정을 참조하세요.
요청 생성
이제 Vision API 서비스가 준비되었으므로 서비스에 대한 요청을 생성할 수 있습니다.
이 코드 스니펫은 다음 작업을 수행합니다.
ImageAnnotatorClient
인스턴스를 클라이언트로 생성합니다.- 로컬 파일 또는 URI로
Image
객체를 생성합니다. Image
객체를 클라이언트의web_detection
메서드로 전달합니다.- 주석을 반환합니다.
응답 출력
작업이 완료되면 WebDetection을 진행하면서 주석에 포함된 항목과 URL을 출력합니다. 다음 섹션에서는 각 주석 유형의 최상위 결과 2개를 보여줍니다.
애플리케이션 실행
애플리케이션을 실행하려면 다음 자동차 이미지의 URL(http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg)을 전달합니다.
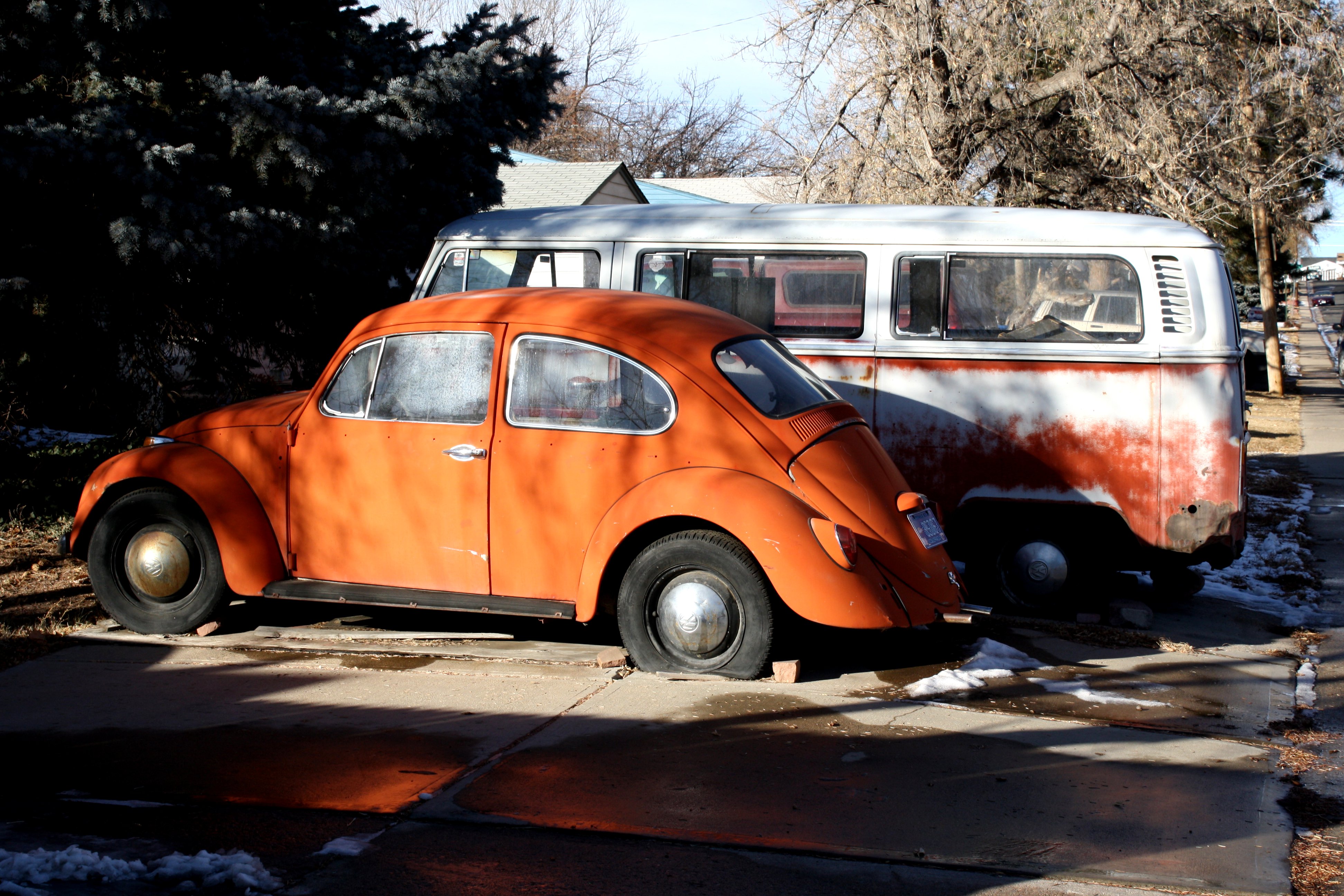
다음은 자동차 이미지의 웹 URL을 전달하는 Python 명령 및 콘솔 출력입니다. 나열된 항목 뒤에 관련성 점수가 추가되었습니다. 정규화되지 않은 점수이므로 서로 다른 이미지 쿼리 간에 비교할 수는 없습니다.
python web_detect.py "http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg"
5 Pages with matching images retrieved Url : http://www.photos-public-domain.com/2011/01/07/old-volkswagen-bug-and-van/ Url : http://pix-hd.com/old+volkswagen+van+for+sale ... 2 Full Matches found: Url : http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg Url : http://www.wbwagen.com/media/old-volkswagen-bug-and-van-picture-free-photograph-photos-public_s_66f487042adad5a6.jpg 4 Partial Matches found: Url : http://www.photos-public-domain.com/wp-content/uploads/2011/01/old-vw-bug-and-van.jpg Url : http://www.wbwagen.com/media/old-vw-bug-and-vanjpg_s_ac343d7f041b5f8d.jpg ... 5 Web entities found: Score : 5.35028934479 Description: Volkswagen Beetle Score : 1.43998003006 Description: Volkswagen Score : 0.828279972076 Description: Volkswagen Type 2 Score : 0.75271999836 Description: Van Score : 0.690039992332 Description: Car
수고하셨습니다. Vision API를 사용하여 웹 감지를 수행했습니다.