Audiens
Tujuan tutorial ini adalah untuk membantu Anda mengembangkan aplikasi menggunakan fitur Petunjuk Crop Vision API. Hal ini mengasumsikan bahwa Anda sudah terbiasa dengan konstruksi dan teknik pemrograman dasar. Namun, meskipun Anda adalah programmer pemula, Anda dapat mengikuti dan menjalankan tutorial ini dengan mudah. Setelah itu, gunakan dokumentasi referensi Vision API untuk membuat aplikasi dasar.
Tutorial ini menjelaskan tentang aplikasi Vision API, yang menunjukkan cara melakukan panggilan ke Vision API untuk menggunakan fitur Petunjuk Crop-nya.
Prasyarat
- Siapkan project Vision API di konsol Google Cloud.
Siapkan lingkungan Anda untuk menggunakan Kredensial Default Aplikasi.
Python
- Instal Python.
- Instal pip.
- Instal Library Klien Google Cloud.
- Menginstal Python Imaging Library
Ringkasan
Tutorial ini membahas aplikasi Vision API dasar yang menggunakan permintaan Crop Hints
. Anda dapat memberikan image untuk diproses melalui URI Cloud Storage (lokasi bucket Cloud Storage) atau disematkan dalam permintaan. Respons Crop Hints
yang berhasil akan menampilkan koordinat untuk
kotak pembatas yang dipangkas di sekitar objek atau wajah yang dominan dalam gambar.
Daftar kode
Saat membaca kode, sebaiknya Anda mengikuti dengan melihat referensi Python Cloud Vision API Python.
Jelajahi lebih lanjut
Mengimpor library
Kami mengimpor library standar:
argparse
untuk mengizinkan aplikasi menerima nama file input sebagai argumenio
untuk file I/O
Impor lainnya:
- Class
ImageAnnotatorClient
dalam librarygoogle.cloud.vision
untuk mengakses Vision API. - Modul
types
dalam librarygoogle.cloud.vision
untuk membuat permintaan - Modul
Image
danImageDraw
dariPython Imaging Library
(PIL). untuk menggambar kotak batas pada gambar input.
Menjalankan aplikasi
Di sini, kita hanya mengurai argumen yang diteruskan yang menentukan nama file gambar lokal, dan meneruskannya ke fungsi untuk memangkas gambar atau menggambar petunjuk.
Mengautentikasi ke API
Sebelum berkomunikasi dengan layanan Vision API, Anda harus
mengautentikasi layanan Anda menggunakan kredensial yang diperoleh sebelumnya. Dalam
aplikasi, cara termudah untuk mendapatkan kredensial adalah dengan menggunakan
Kredensial Default Aplikasi
(ADC). Secara default, library klien akan mencoba mendapatkan kredensial dari variabel lingkungan GOOGLE_APPLICATION_CREDENTIALS
, yang harus ditetapkan agar mengarah ke file kunci JSON akun layanan Anda (lihat Siapkan Akun Layanan
untuk mengetahui informasi selengkapnya.)
Mendapatkan anotasi petunjuk pemangkasan untuk gambar
Setelah library klien Vision diautentikasi, kita dapat mengakses layanan dengan memanggil metode crop_hints
dari instance ImageAnnotatorClient
.
Rasio aspek untuk output ditentukan dalam
objek ImageContext
; jika beberapa rasio aspek diteruskan, beberapa
petunjuk crop akan ditampilkan, satu untuk setiap rasio aspek.
Library klien melakukan enkapsulasi detail permintaan dan respons ke API. Lihat Reference Vision API untuk mengetahui informasi lengkap tentang struktur permintaan.
Menggunakan respons untuk memangkas atau menggambar kotak pembatas petunjuk
Setelah operasi berhasil diselesaikan, respons API akan berisi koordinat kotak pembatas dari satu atau beberapa cropHint
. Metode
draw_hint
menggambar garis di sekitar kotak pembatas CropHints, lalu menulis
gambar ke output-hint.jpg
.
Metode crop_to_hint
akan memangkas gambar menggunakan petunjuk pemangkasan yang disarankan.
Menjalankan aplikasi
Untuk menjalankan aplikasi, Anda dapat
mendownload file cat.jpg
ini
(Anda mungkin perlu mengklik kanan link),
lalu meneruskan lokasi tempat Anda mendownload di komputer lokal Anda
ke aplikasi tutorial (crop_hints.py
).
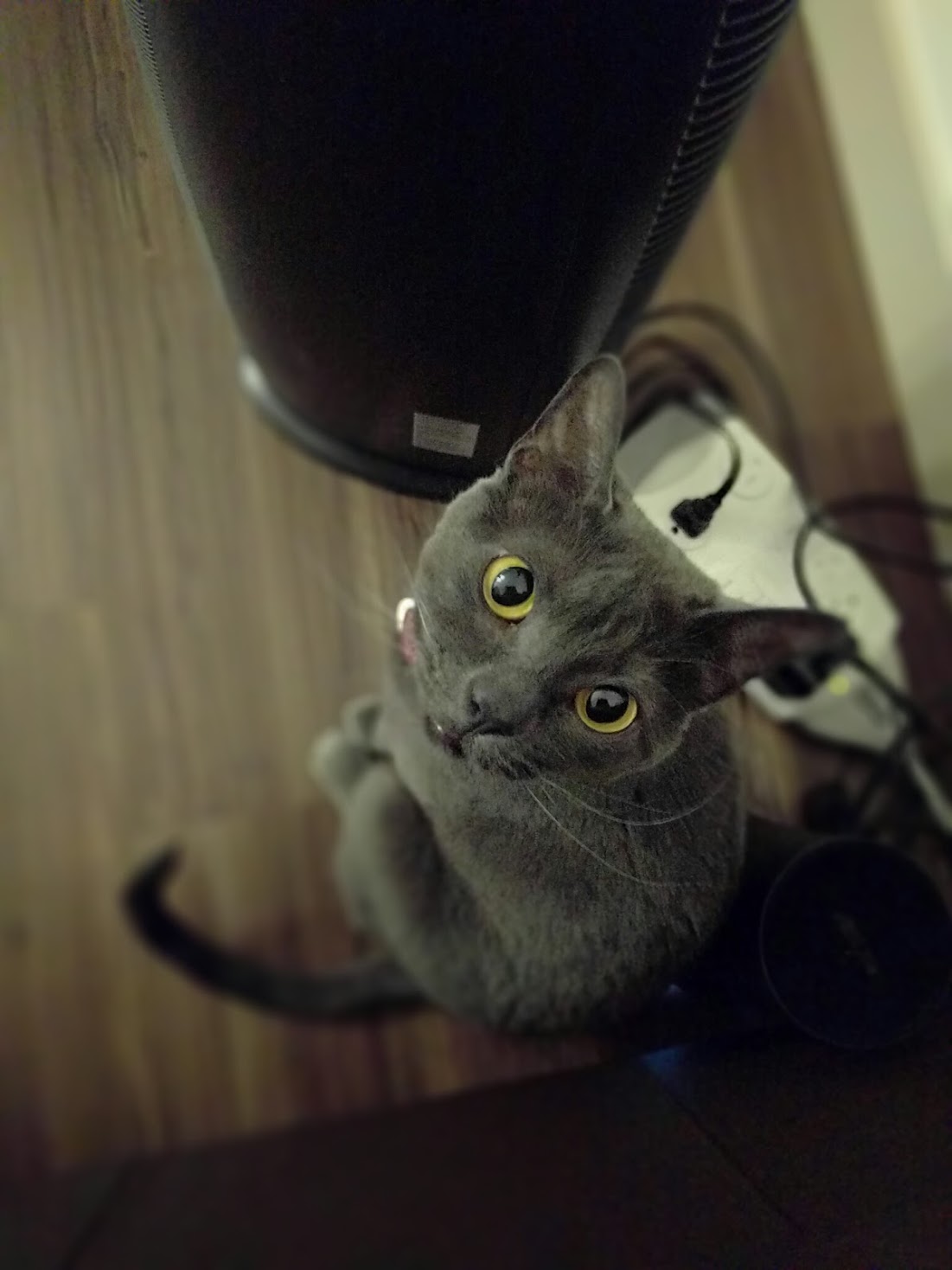
Berikut adalah perintah Python, diikuti dengan output konsol, yang menampilkan
respons cropHintsAnnotation
JSON. Respons ini menyertakan koordinat
kotak pembatas cropHints
. Kami meminta area pemangkasan dengan rasio aspek lebar-tinggi 1,77, dan koordinat x,y kiri atas, kanan bawah,
untuk kotak pangkas yang ditampilkan adalah 0,336
, 1100,967
singkat ini.
python crop_hints.py cat.jpeg crop
{ "responses": [ { "cropHintsAnnotation": { "cropHints": [ { "boundingPoly": { "vertices": [ { "y": 336 }, { "x": 1100, "y": 336 }, { "x": 1100, "y": 967 }, { "y": 967 } ] }, "confidence": 0.79999995, "importanceFraction": 0.69 } ] } } ] }
Dan berikut adalah gambar yang dipangkas.
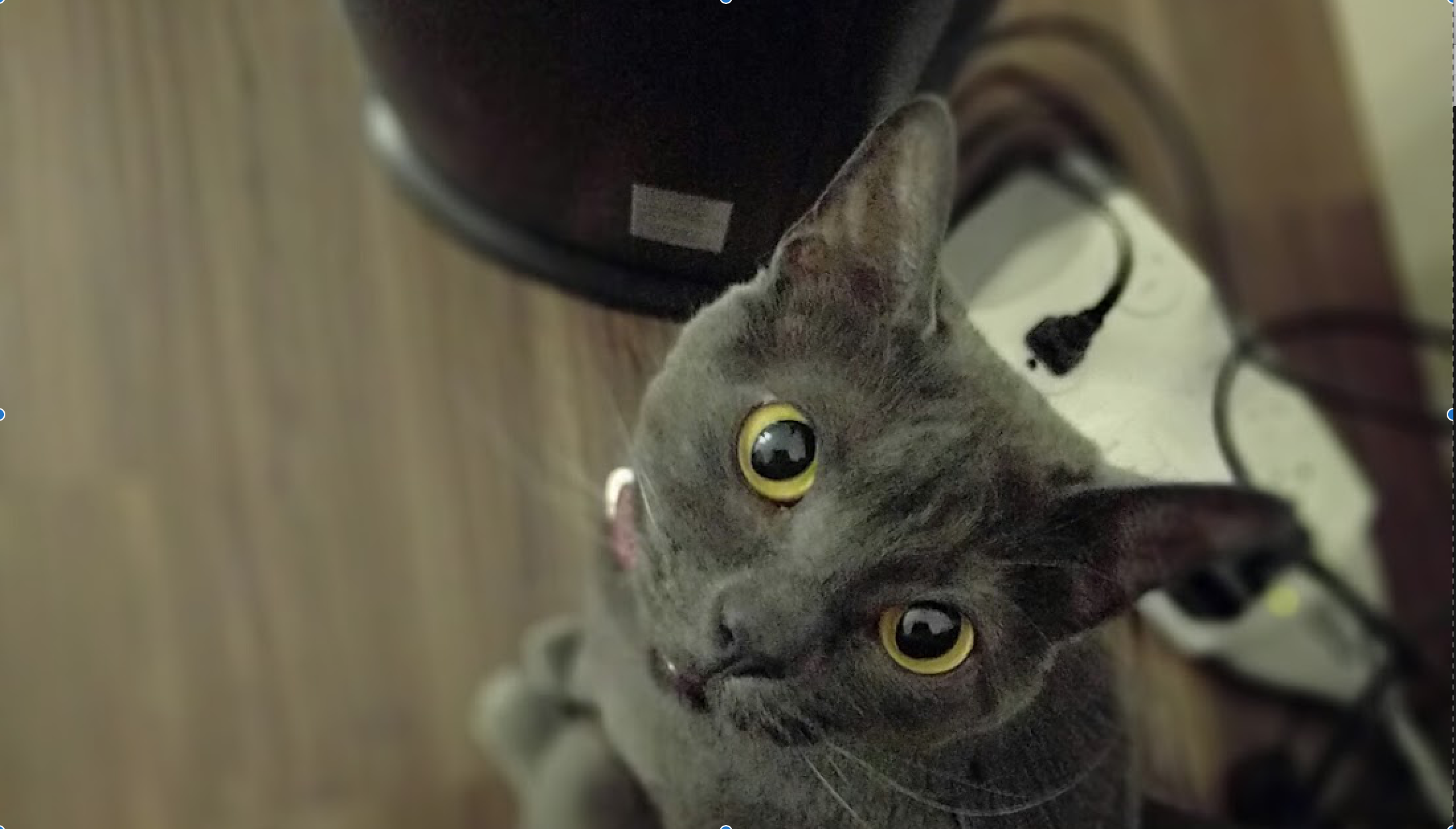
Selamat! Anda telah menjalankan Cloud Vision Crop Hints API untuk menampilkan koordinat kotak pembatas yang dioptimalkan di sekitar objek dominan yang terdeteksi dalam gambar.