CA プールの削除
このページでは、CA プールを削除する方法について説明します。
CA プールを削除できるのは、CA プール内の CA が完全に削除された後のみです。CA Service は、削除プロセスが開始されてから 30 日間の猶予期間の後に、CA を完全に削除します。詳細については、CA を削除するをご覧ください。
CA プールを削除するには、次の手順を行います。
コンソール
- Google Cloud コンソールの [Certificate Authority Service] ページに移動します。
- [CA プール マネージャー] タブをクリックします。
- CA プールのリストで、削除する CA プールを選択します。
- [削除] をクリックします。
- 表示されたダイアログ ボックスで [確認] をクリックします。
Certificate Authority Service に移動
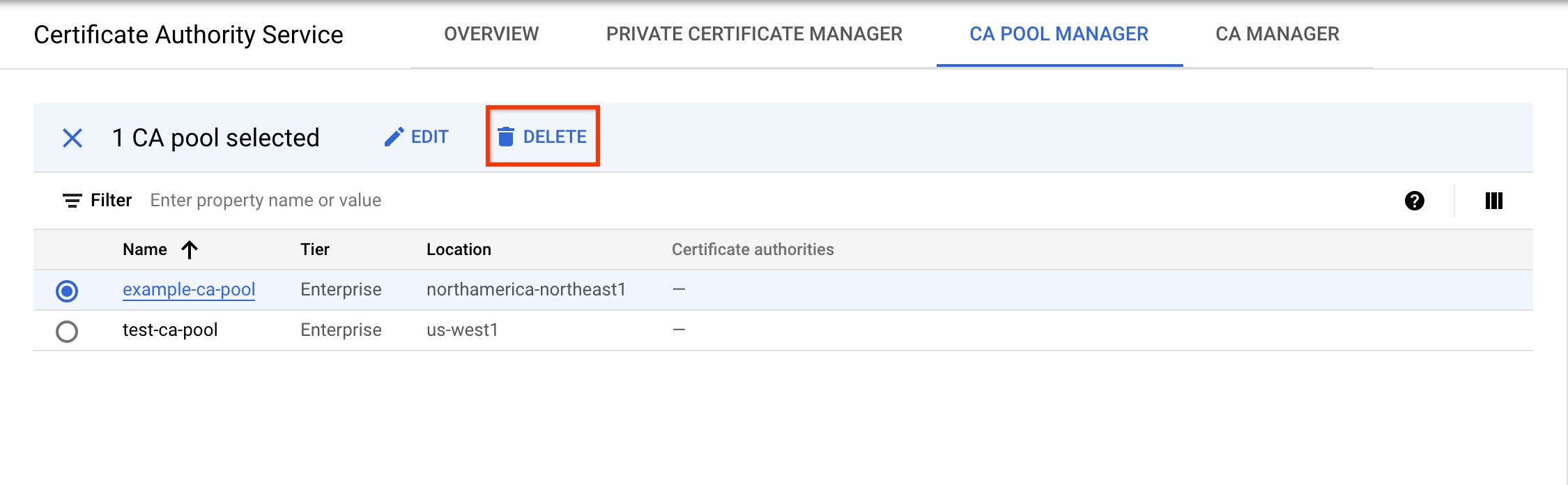
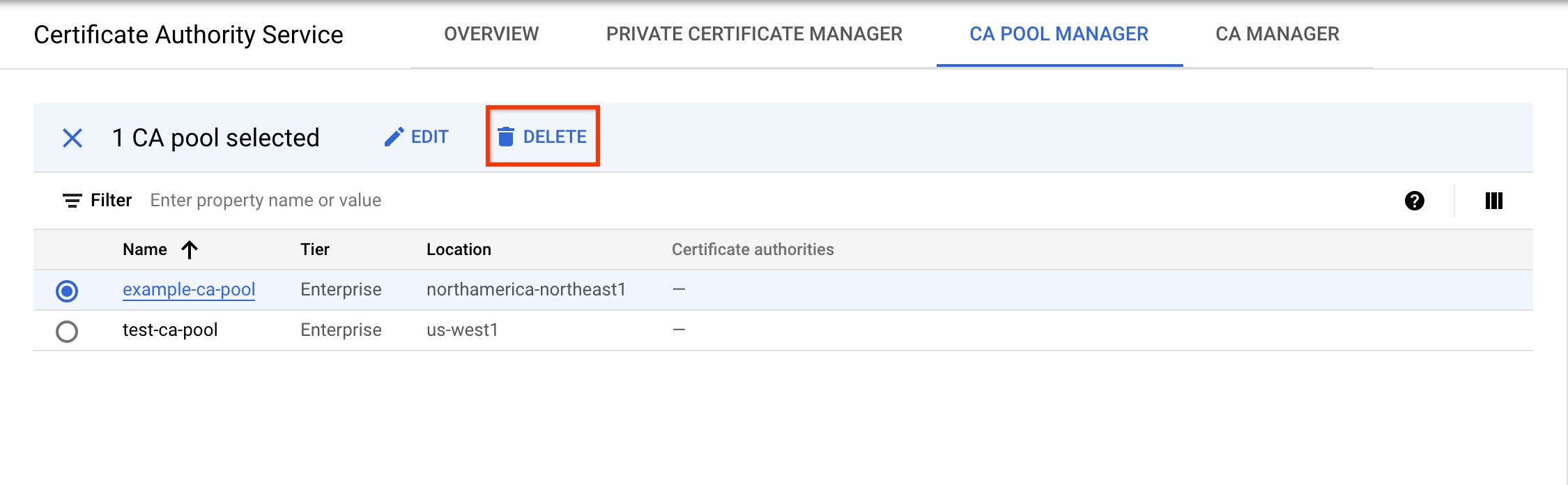
gcloud
次のコマンドを実行します。
gcloud privateca pools delete POOL_ID
POOL_ID は、削除する CA プールの名前に置き換えます。
gcloud privateca pools delete
コマンドの詳細については、gcloud privateca pools delete をご覧ください。
Go
CA Service への認証を行うには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Java
CA Service への認証を行うには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Python
CA Service への認証を行うには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
次のステップ
- 発行ポリシーを使用して CA プールが発行できる証明書のタイプを制限する方法を学習する。
- 認証局を更新する方法を学習する。
- 認証局を削除する方法を学習する。