認証局を削除する
Certificate Authority Service を使用すると、既存の認証局(CA)を削除できます。CA は、削除プロセスが開始されてから 30 日間の猶予期間が経過すると、完全に削除されます。猶予期間が経過すると、CA Service は CA と、ネストされたすべてのアーティファクト(証明書や証明書失効リスト(CRL)など)を完全に削除します。
削除された CA によって使用されていた顧客管理の Google Cloud リソース(Cloud Storage バケットや Cloud Key Management Service 鍵など)は削除されません。Google 管理のリソースと顧客管理のリソースの詳細については、リソースの管理をご覧ください。
削除された CA は猶予期間中は課金されません。ただし、CA を復元すると、CA が DELETED
状態であった間、CA の課金ティアで課金されます。
準備
CA Service オペレーション マネージャー(
roles/privateca.caManager
)または CA Service 管理者(roles/privateca.admin
)の Identity and Access Management(IAM)ロールがあることを確認します。CA Service の事前定義された IAM ロールの詳細については、IAM を使用したアクセス制御をご覧ください。IAM ロールの付与については、単一のロールを付与するをご覧ください。
CA が次の条件を満たしていることを確認します。
- CA は、
AWAITING_USER_ACTIVATION
、DISABLED
、またはSTAGED
の状態である必要があります。詳細については、認証局の状態をご覧ください。
- CA に有効な証明書を含めることはできません。CA を完全に削除する前に、CA によって発行された証明書を取り消すことをおすすめします。CA を完全に削除した後、有効な証明書を取り消すことはできません。
- CA は、
CA を削除する
CA の削除を開始するには、次の手順を行います。
Console
- Google Cloud コンソールの [Certificate Authority Service] ページに移動します。
- [CA マネージャー] タブをクリックします。
- CA のリストで、削除する CA を選択します。
- [無効にする] をクリックします。
- 表示されたダイアログで [登録解除] をクリックします。
- [削除] をクリックします。
- 表示されたダイアログで [登録解除] をクリックします。
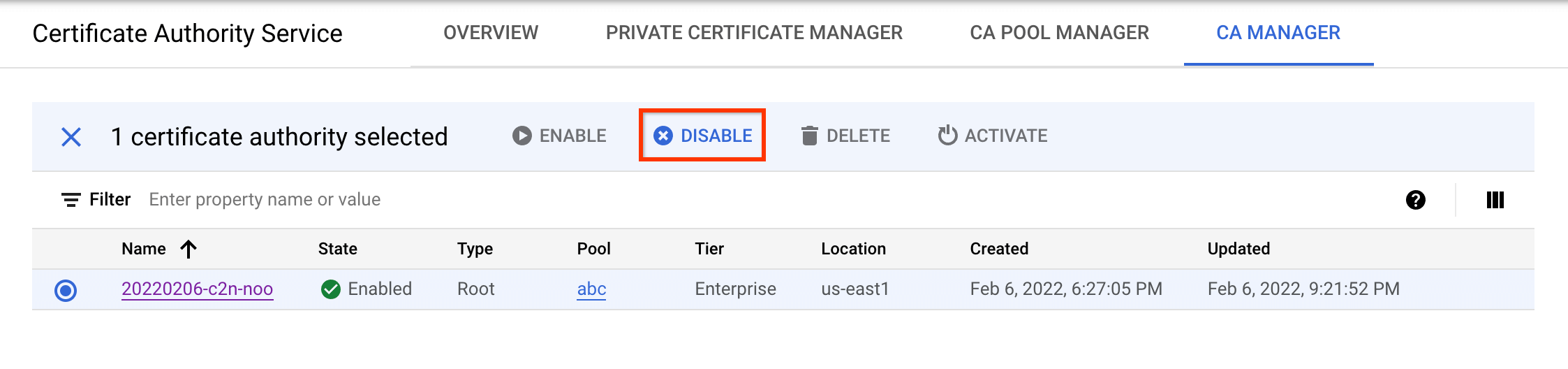
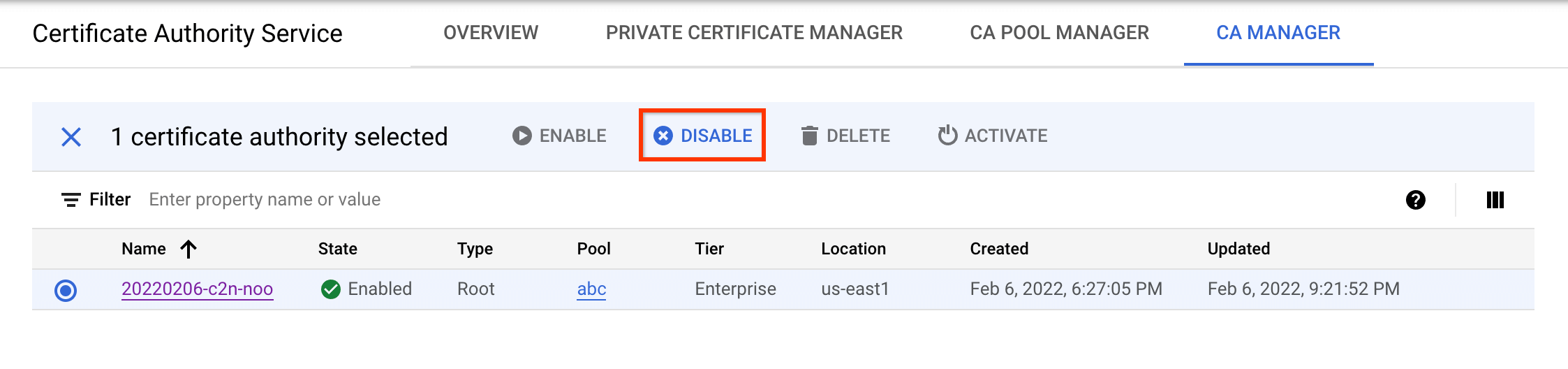
gcloud
CA の状態をチェックして、無効になっていることを確認します。削除できるのは、
DISABLED
状態の CA のみです。gcloud privateca roots describe CA_ID --pool=POOL_ID \ --format="value(state)"
以下を置き換えます。
- CA_ID: CA の固有識別子。
- POOL_ID: CA を含む CA プールの名前。
gcloud privateca roots describe
コマンドの詳細については、gcloud privateca roots describe をご覧ください。CA が無効になっていない場合は、次のコマンドを実行して CA を無効にします。
gcloud privateca roots disable CA_ID --pool=POOL_ID
gcloud privateca roots disable
コマンドの詳細については、gcloud privateca roots disable をご覧ください。CA を削除します。
gcloud privateca roots delete CA_ID --pool=POOL_ID
CA に有効な証明書があっても、
gcloud
コマンドで--ignore-active-certificates
フラグを指定すると CA を削除できます。gcloud privateca roots delete
コマンドの詳細については、gcloud privateca roots delete をご覧ください。プロンプトが表示されたら、CA を削除することを確認します。
確認後、CA が削除されるようにスケジュールされ、30 日間の猶予期間が開始します。このコマンドは、CA が削除される予定の日時を出力します。
Deleted Root CA [projects/PROJECT_ID/locations/us-west1/caPools/POOL_ID/certificateAuthorities/CA_ID] can be undeleted until 2020-08-14T19:28:39Z.
Go
CA Service への認証を行うには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Java
CA Service への認証を行うには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Python
CA Service への認証を行うには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
削除された CA の有効期限を確認する
CA が完全に削除される日時を確認するには、次の操作を行います。
Console
- [CA プール マネージャー] タブをクリックします。
- 削除した CA を含む CA プールの名前をクリックします。
CA の有効期限は、[CA プール] ページのテーブルで確認できます。
gcloud
CA の予想削除時間を確認するには、次のコマンドを実行します。
gcloud privateca roots describe CA_ID \
--pool=POOL_ID \
--format="value(expireTime.date())"
以下を置き換えます。
- CA_ID: CA の名前。
- POOL_ID: CA を含む CA プールの名前。
このコマンドは、CA Service が CA を削除する予想日時を返します。
2020-08-14T19:28:39
CA が完全に削除されたことを確認するには、次のコマンドを実行します。
gcloud privateca roots describe CA_ID --pool=POOL_ID
CA が正常に削除されると、コマンドから次のエラーが返されます。
ERROR: (gcloud.privateca.roots.describe) NOT_FOUND: Resource 'projects/PROJECT_ID/locations/LOCATION/caPools/POOL_ID/certificateAuthorities/CA_ID' was not found