機密データの保護では、画像内の機密データを検出して分類できます。 機密データの保護は、infoType 検出器と光学式文字認識(OCR)を使用して、テキストを Base64 でエンコードした画像を検査し、テキスト内の機密データを検出します。そして、検出した機密データの場所を返します。
入力として画像を指定すると、機密データの保護は画像内の機密データを検出します。検査オペレーションの出力には、検出された infoType、一致の可能性、機密データの保護が機密データを検出した領域を示すピクセル座標と長さの値が含まれます。画像の左下隅の座標は (0,0)
です。
画像にあるすべてのデフォルトの infoType を検査する
画像に含まれる機密データを検査するには、base64 でエンコードされた画像を DLP API の content.inspect
メソッドに送信します。検索する特定の情報タイプ(infoTypes)を指定しない限り、機密データの保護は最も一般的な infoType を検索します。
画像にあるデフォルトの infoType を検査するには:
- 画像を base64 でエンコードします。
- DLP API の
content.inspect
メソッドにリクエストを送信します。デフォルトの infoType を検査する場合、リクエストには base64 エンコードの画像のみが含まれている必要があります。
たとえば、次の画像について考えてみます。この画像は、紙のドキュメントをスキャンして生成された一般的な画像ファイルの例です。

この画像のデフォルトの infoType を検査するには、次のリクエストを DLP API の content.inspect
メソッドに送信します。
C#
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Go
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Java
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Node.js
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
PHP
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Python
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
REST
{ "item": { "byteItem": { "data": "[BASE64-ENCODED-IMAGE]", "type": "IMAGE_JPEG" } } }
機密データの保護は、以下を返します。
{ "result": { "findings": [ { "infoType": { "name": "PHONE_NUMBER" }, "likelihood": "UNLIKELY", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 98, "left": 986, "width": 102, "height": 117 }, { "top": 98, "left": 1092, "width": 29, "height": 114 }, { "top": 95, "left": 1111, "width": 82, "height": 115 }, { "top": 95, "left": 1197, "width": 29, "height": 114 }, { "top": 90, "left": 1203, "width": 185, "height": 118 } ] } } ] }, "createTime": "2019-11-01T22:07:05.870Z" }, { "infoType": { "name": "US_SOCIAL_SECURITY_NUMBER" }, "likelihood": "VERY_LIKELY", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 98, "left": 986, "width": 102, "height": 117 }, { "top": 98, "left": 1092, "width": 29, "height": 114 }, { "top": 95, "left": 1111, "width": 82, "height": 115 }, { "top": 95, "left": 1197, "width": 29, "height": 114 }, { "top": 90, "left": 1203, "width": 185, "height": 118 } ] } } ] }, "createTime": "2019-11-01T22:07:05.871Z" }, { "infoType": { "name": "EMAIL_ADDRESS" }, "likelihood": "LIKELY", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 340, "left": 334, "width": 57, "height": 58 }, { "top": 340, "left": 384, "width": 12, "height": 58 }, { "top": 340, "left": 387, "width": 79, "height": 59 }, { "top": 341, "left": 467, "width": 12, "height": 58 }, { "top": 340, "left": 476, "width": 119, "height": 61 }, { "top": 341, "left": 589, "width": 12, "height": 58 }, { "top": 342, "left": 592, "width": 45, "height": 58 } ] } } ] }, "createTime": "2019-11-01T22:07:05.869Z" }, { "infoType": { "name": "PHONE_NUMBER" }, "likelihood": "POSSIBLE", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 394, "left": 335, "width": 50, "height": 77 }, { "top": 394, "left": 380, "width": 17, "height": 77 }, { "top": 394, "left": 387, "width": 51, "height": 77 }, { "top": 394, "left": 433, "width": 17, "height": 77 }, { "top": 394, "left": 436, "width": 77, "height": 77 } ] } } ] }, "createTime": "2019-11-01T22:07:05.870Z" }, { "infoType": { "name": "DATE" }, "likelihood": "LIKELY", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 572, "left": 1129, "width": 71, "height": 38 } ] } } ] }, "createTime": "2019-11-01T22:07:05.921Z" } ] } }
機密データの保護では次の infoType が検出されましたが、それぞれの一致の可能性は異なります。
- 電話番号(一致する可能性が低い)
- 米国社会保障番号(一致する可能性が非常に高い)
- メールアドレス(一致する可能性が高い)
- 電話番号(一致する可能性がある)
- 日付(一致する可能性が高い)
画像に各境界ボックスを描画すると、次のようになります。機密データの保護では、1 つの機密データのインスタンスが画像内のどこにあるかを示すために、複数のボックスが使われることが多いことに注意してください。
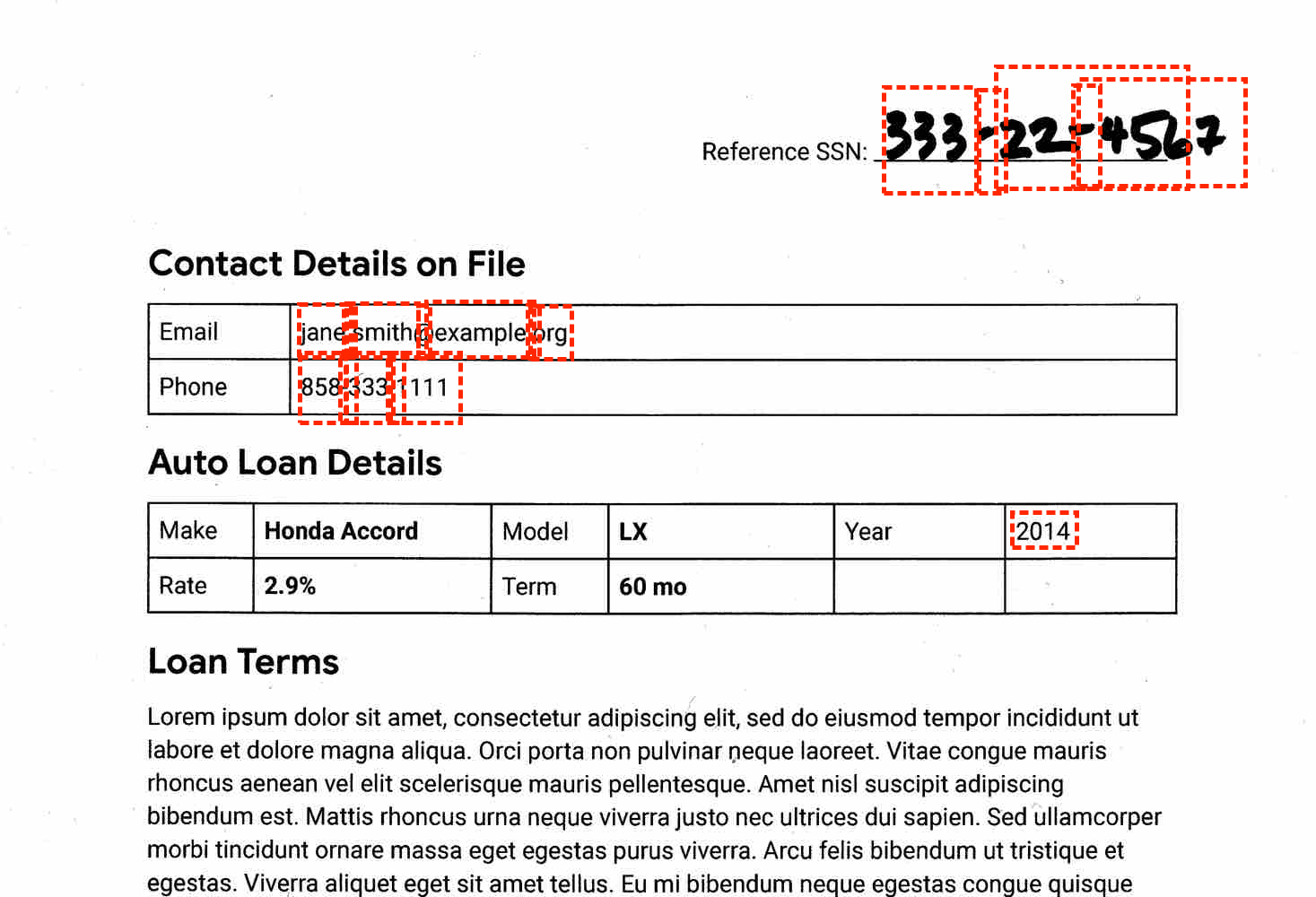
手書きの社会保障番号、メールアドレス、電話番号を検出することに加えて、機密データの保護は年も検出していることに注目してください。これが最適な動作であるとは限らないため、次の例で、特定の infoType のみを検査する方法を説明します。
画像にある特定の infoType を検査する
特定の機密データ タイプに対してのみ画像を検査するには、対応する組み込みの infoType を指定します。
画像にある特定の infoType を検査するには:
- 画像を base64 でエンコードします。
- DLP API の
content.inspect
メソッドにリクエストを送信します。リクエストには以下を含める必要があります。- base64 でエンコードされた画像
- 1 つ以上の infoType 検出器
前のセクションの元の画像について考えてみます。米国社会保障番号、メールアドレス、電話番号のみを検査するには、次の JSON を DLP API の content.inspect
メソッドに送信します。
C#
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Go
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Java
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Node.js
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
PHP
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Python
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
REST
{ "item": { "byteItem": { "data": "[BASE64-ENCODED-IMAGE]", "type": "IMAGE_JPEG" } }, "inspectConfig": { "infoTypes": [ { "name": "US_SOCIAL_SECURITY_NUMBER" }, { "name": "PHONE_NUMBER" }, { "name": "EMAIL_ADDRESS" } ] } }
機密データの保護は、以下を返します。
{ "result": { "findings": [ { "infoType": { "name": "US_SOCIAL_SECURITY_NUMBER" }, "likelihood": "VERY_LIKELY", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 98, "left": 986, "width": 102, "height": 117 }, { "top": 98, "left": 1092, "width": 29, "height": 114 }, { "top": 95, "left": 1111, "width": 82, "height": 115 }, { "top": 95, "left": 1197, "width": 29, "height": 114 }, { "top": 90, "left": 1203, "width": 185, "height": 118 } ] } } ] }, "createTime": "2019-11-01T22:58:56.985Z" }, { "infoType": { "name": "EMAIL_ADDRESS" }, "likelihood": "LIKELY", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 340, "left": 334, "width": 57, "height": 58 }, { "top": 340, "left": 384, "width": 12, "height": 58 }, { "top": 340, "left": 387, "width": 79, "height": 59 }, { "top": 341, "left": 467, "width": 12, "height": 58 }, { "top": 340, "left": 476, "width": 119, "height": 61 }, { "top": 341, "left": 589, "width": 12, "height": 58 }, { "top": 342, "left": 592, "width": 45, "height": 58 } ] } } ] }, "createTime": "2019-11-01T22:58:56.984Z" }, { "infoType": { "name": "PHONE_NUMBER" }, "likelihood": "POSSIBLE", "location": { "contentLocations": [ { "imageLocation": { "boundingBoxes": [ { "top": 394, "left": 335, "width": 50, "height": 77 }, { "top": 394, "left": 380, "width": 17, "height": 77 }, { "top": 394, "left": 387, "width": 51, "height": 77 }, { "top": 394, "left": 433, "width": 17, "height": 77 }, { "top": 394, "left": 436, "width": 77, "height": 77 } ] } } ] }, "createTime": "2019-11-01T22:58:56.985Z" } ] } }
出力からわかるように、機密データの保護は、米国社会保障番号、メールアドレス、電話番号を検出しました。
JSON で DLP API を使用する方法については、JSON クイックスタートをご覧ください。
コードの例
次の複数の言語のサンプルコードは、機密データの保護を使用して、機密データに関して画像を検査する方法を示しています。
C#
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Go
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Java
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Node.js
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
PHP
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
Python
機密データの保護用のクライアント ライブラリをインストールして使用する方法については、機密データの保護のクライアント ライブラリをご覧ください。
機密データの保護のために認証するには、アプリケーションのデフォルト認証情報を設定します。 詳細については、ローカル開発環境の認証の設定をご覧ください。
試してみる
content.inspect
のリファレンス ページの API Explorer で、このページの各例を実際に試すことができます。または、独自の画像で試すこともできます。
次のステップ
- 画像の検査と秘匿化の詳細を確認する。
- 画像内の機密データを秘匿化する方法を確認する。