Menghapus kumpulan CA
Halaman ini menjelaskan cara menghapus kumpulan CA.
Anda dapat menghapus kumpulan CA hanya setelah Anda menghapus secara permanen semua CA dalam kumpulan CA tersebut. Layanan CA menghapus CA secara permanen setelah masa tenggang 30 hari sejak proses penghapusan dimulai. Untuk mengetahui informasi selengkapnya, lihat Menghapus CA.
Untuk menghapus kumpulan CA, gunakan petunjuk berikut.
Konsol
- Buka halaman Certificate Authority Service di Konsol Google Cloud.
- Klik tab Pengelola kumpulan CA.
- Dalam daftar kumpulan CA, pilih kumpulan CA yang ingin Anda hapus.
- Klik Delete.
- Di kotak dialog yang terbuka, klik Konfirmasi.
Buka Certificate Authority Service
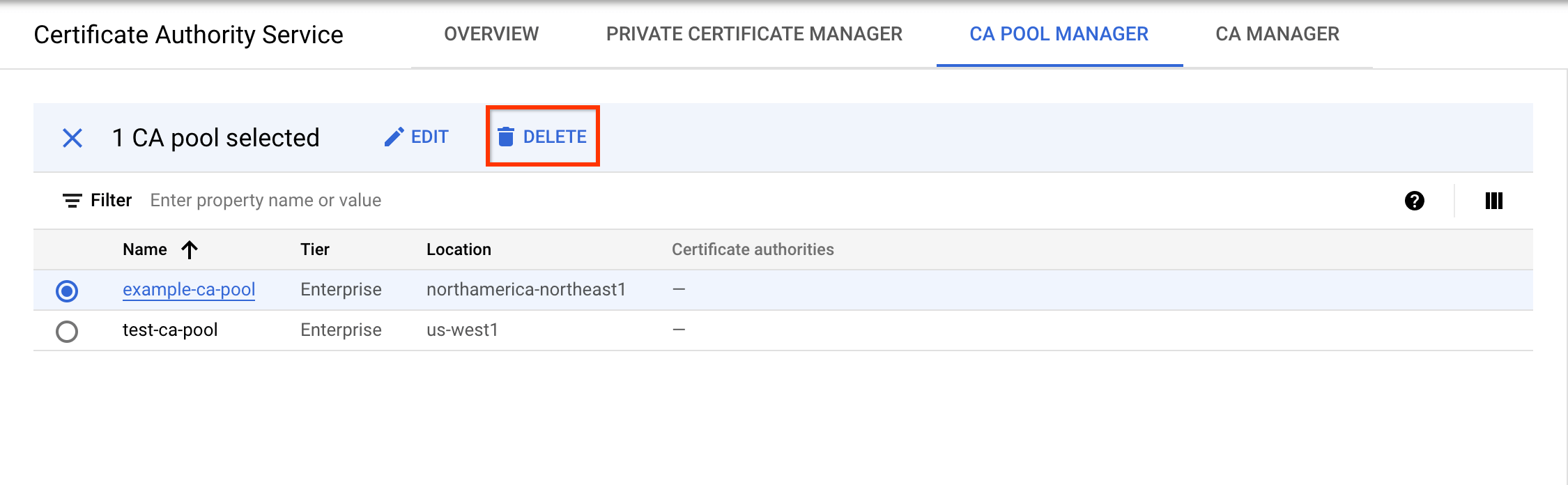
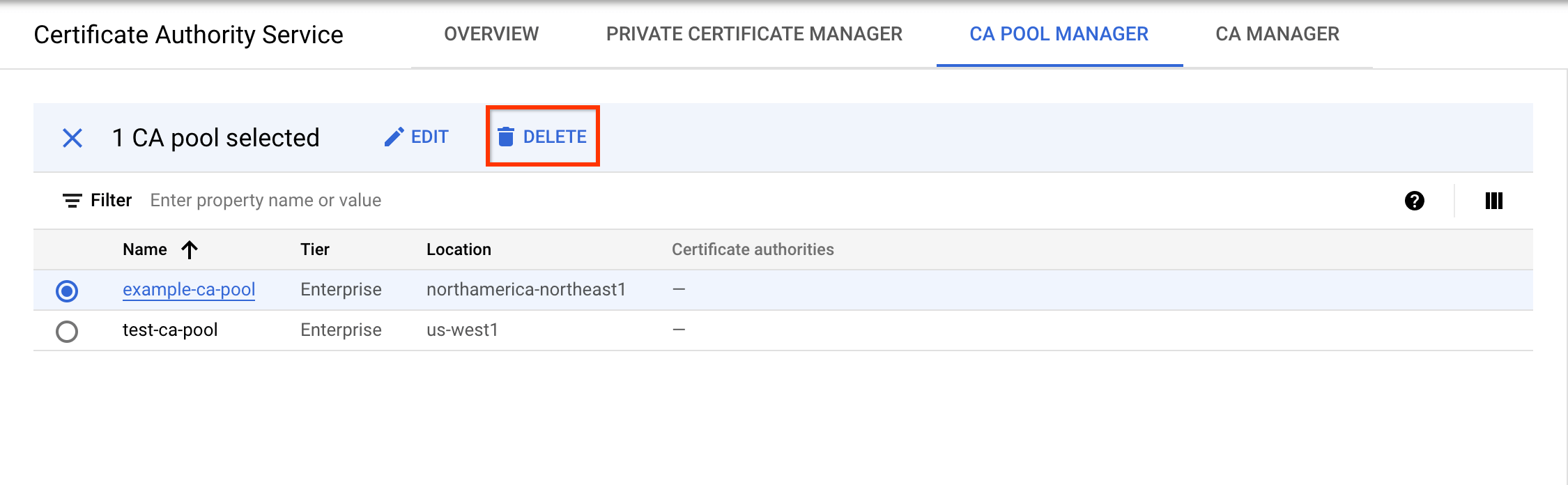
gcloud
Jalankan perintah berikut:
gcloud privateca pools delete POOL_ID
Ganti POOL_ID dengan nama kumpulan CA yang ingin Anda hapus.
Untuk mengetahui informasi selengkapnya tentang perintah gcloud privateca pools delete
, lihat gcloud privateca pool delete.
Go
Untuk mengautentikasi ke CA Service, siapkan Kredensial Default Aplikasi. Untuk mengetahui informasi selengkapnya, baca Menyiapkan autentikasi untuk lingkungan pengembangan lokal.
Java
Untuk mengautentikasi ke CA Service, siapkan Kredensial Default Aplikasi. Untuk mengetahui informasi selengkapnya, baca Menyiapkan autentikasi untuk lingkungan pengembangan lokal.
Python
Untuk mengautentikasi ke CA Service, siapkan Kredensial Default Aplikasi. Untuk mengetahui informasi selengkapnya, baca Menyiapkan autentikasi untuk lingkungan pengembangan lokal.
Langkah selanjutnya
- Pelajari cara menggunakan kebijakan penerbitan untuk membatasi jenis sertifikat yang dapat diterbitkan oleh kumpulan CA.
- Pelajari cara memperbarui certificate authority.
- Pelajari cara menghapus certificate authority.