Build a Java Spring Boot app in IntelliJ with Gemini assistance
Giovanni Galloro
EMEA Solutions Lead, Application Modernization
Gemini Code Assist offers AI assistance for developers it comes with IDE integrations for Visual Studio Code and JetBrains IDEs, including IntelliJ IDEA.
This article explains how to create a simple Java Spring Boot web application from scratch with the help of Gemini Code Assist inside Jetbrains IntelliJ IDEA.
You will start from an empty project created with Spring Initializr and will experiment how to use Gemini assisted code generation to create your application and add unit tests.
In addition to the instructions below, you can also follow this video walkthrough.
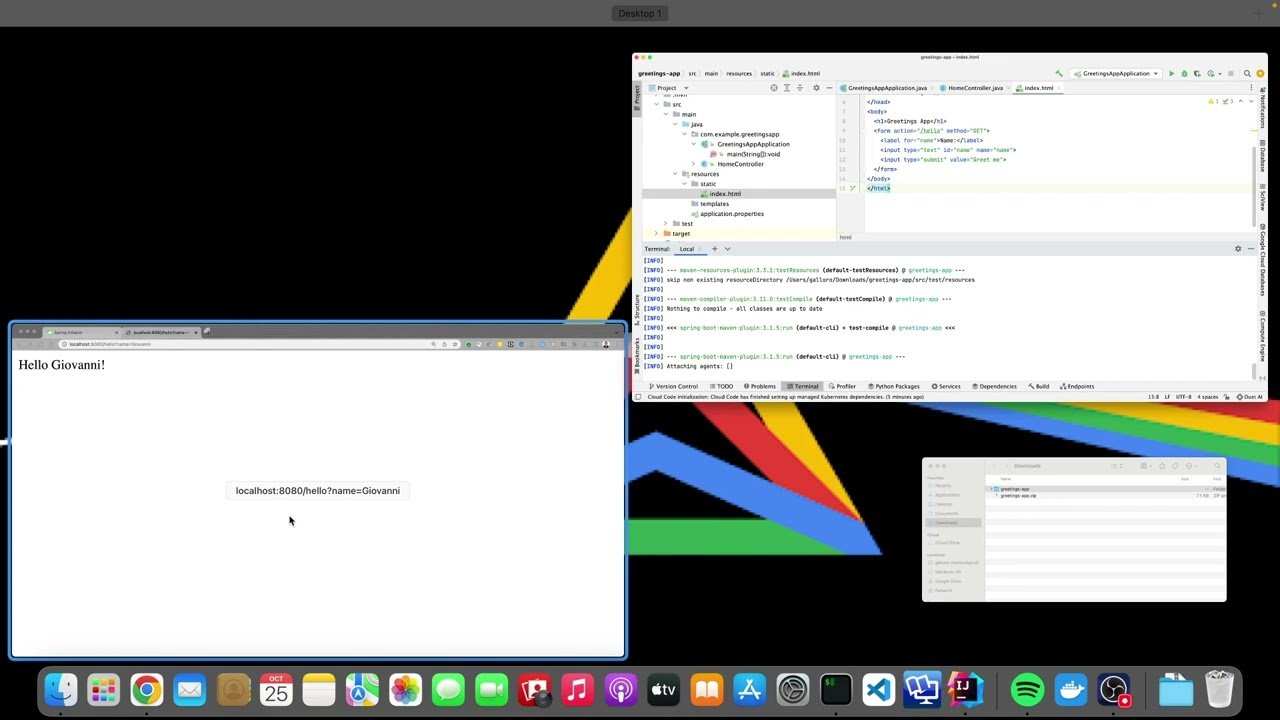
What you need
- A Gemini enabled Google Cloud project where:
- The Gemini for Google Cloud has been enabled
- Billing has been enabled
- A computer with IntelliJ IDEA Community or Ultimate edition installed.
- Cloud Code for IntelliJ plugin installed
- Signed In to Google Cloud from the IDE and with a project selected. If you don’t see the Tools menu in IntelliJ you can open the action menu with Ctrl + Shift + A (Windows) or Cmd + Shift + A (Mac), type “main menu” in the search, and then select Main Menu. From there you can select Tools > Gemini + Google Cloud Code > Sign in to Google Cloud Platform.


Build a starting Spring Boot project
For creating your application you need to start from a sample Spring Boot project created with Spring Initializr, you can do that following the steps below. If you have IntelliJ IDEA Ultimate edition you can also do that directly from the “New” > “Project” flow in the IDE selecting the same options
- Go to https://start.spring.io/
- In the Spring Initializer page, under Project, select Maven
- Select the latest Spring Boot version (not snapshot)
- Under Project Metadata
a. Change Artifact and Name to “greeting-app”
b. Change Description to “Greeting App made with Gemini assistance” - Leave the other options as they are


6. Click on ADD DEPENDENCIES in the higher right corner of the page
7. Select Spring Web from the list
8. Click GENERATE in the bottom of the page, you will be prompted to download the greeting-app.zip file
9. Save the file where it works best for you (in a temporary location if you will delete the project after when you’ll finish) and unzip the file.
Build your code with Gemini code generation assistance
- In IntelliJ go to File > Open (or click Open in the welcome screen)
- Select the folder where you have unzipped your file and click Open
- IntelliJ will open the project folder, double click the GreetingAppApplication class to view its content
- Under src/main/java/com.example.greetingapp, create a new file called HomeController.java
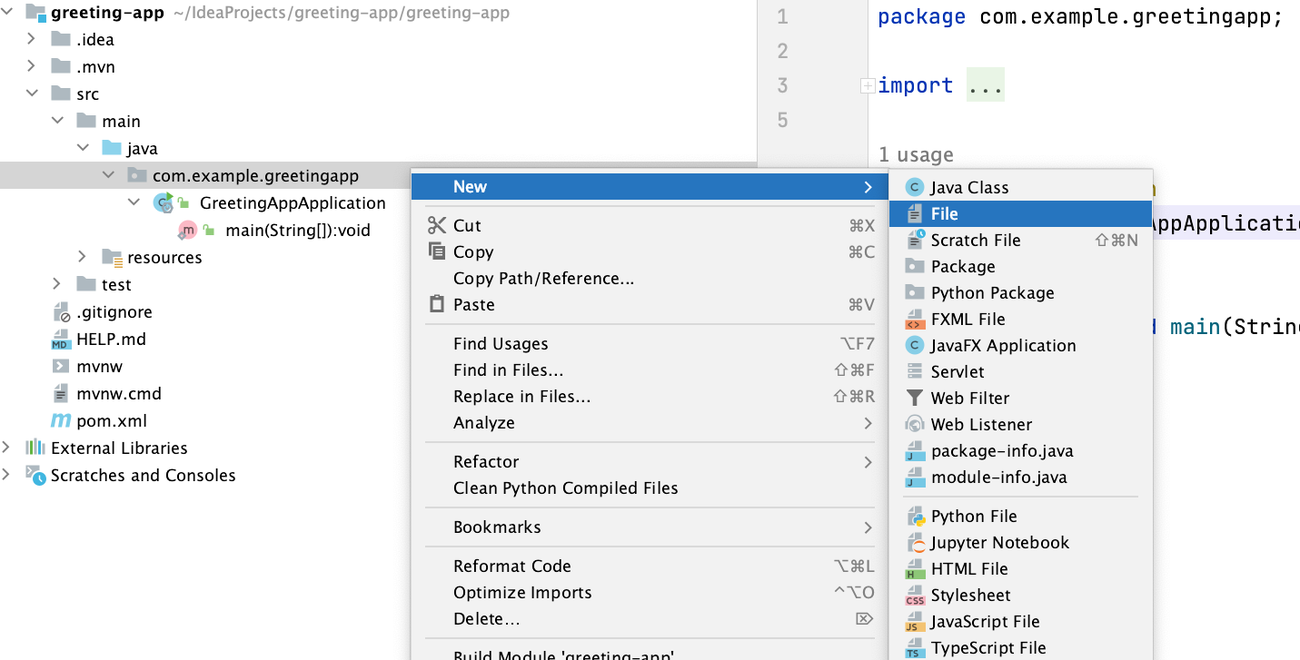
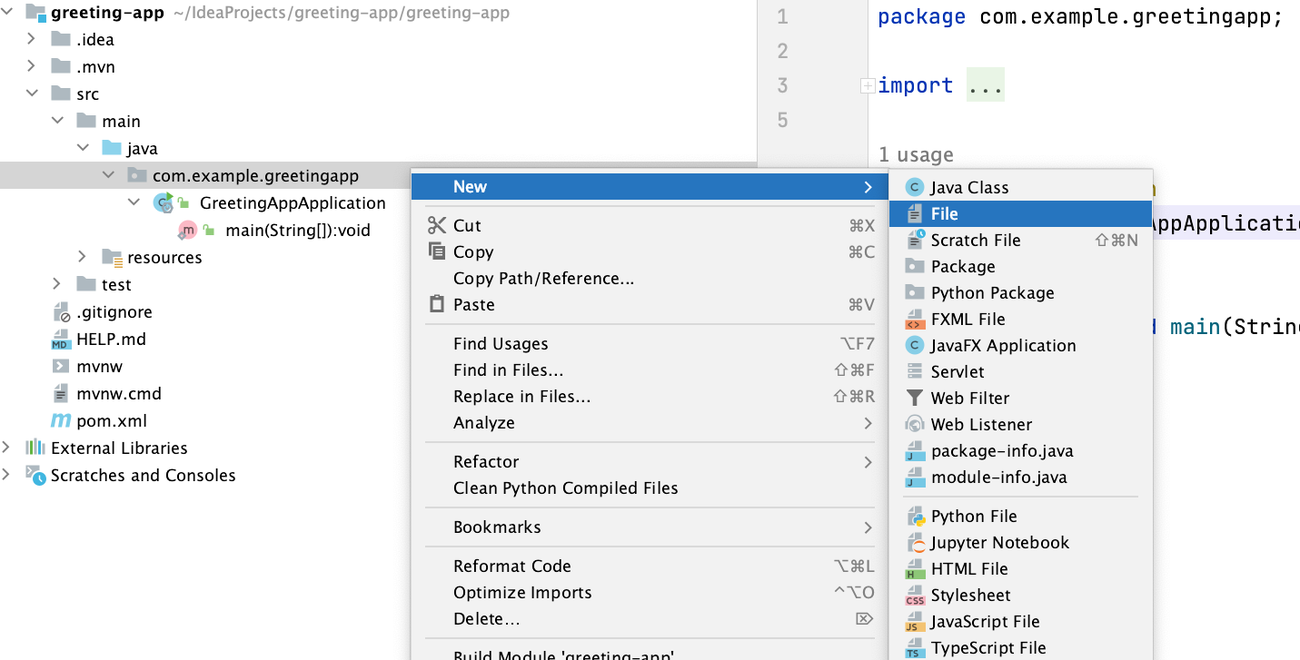
5. At the top of the HomeController.java file type the following comment:
6. Hit Enter and then, to generate code, press Alt+Shift+\ (Windows/Linux) or Option+Shift+\ (macOS). Alternatively you can click on the Gemini icon near the comment line
7. You should get code generated in the form of ghost text. The code should be functionally similar to the following, with a @GetMapping("/hello")
annotation and the hello()
method that greets an user based on the value of the name variable in the request, or uses the value “World” if the variable has no value:
8. If you get the same code as above, hit Tab to accept the suggestion. If code is different you can evaluate if it will work for your goal, decide to make some changes or use the example code above.
9. Probably part of the proposed code will be highlighted and you will have warnings in IntelliJ, you can check them in the problems pane. Gemini Code Assist license checking is warning that the code generated is, at length, taken from one of the samples in the training data so it could be subject to licenses. The warning message includes the source of the training data so that you can check.


10. Now let’s check if generated code works, in the IntelliJ terminal, type:
11. You will see the output of Spring log messages in the terminal.
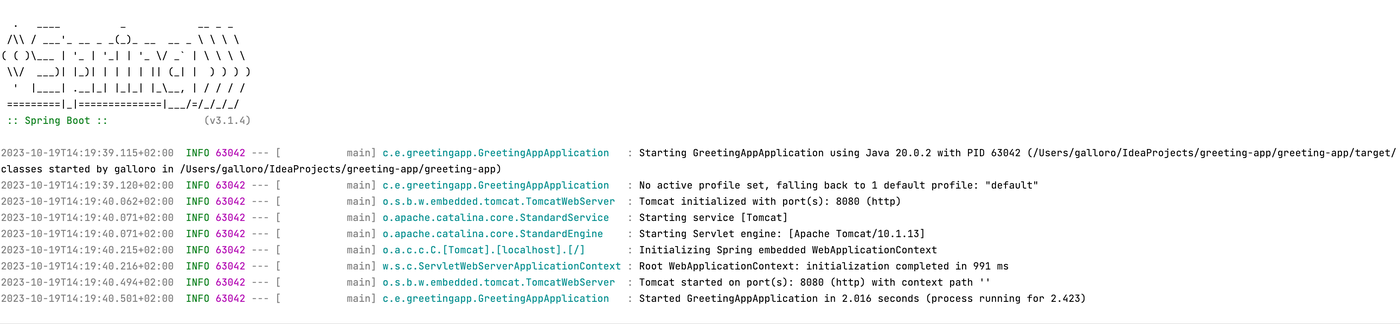
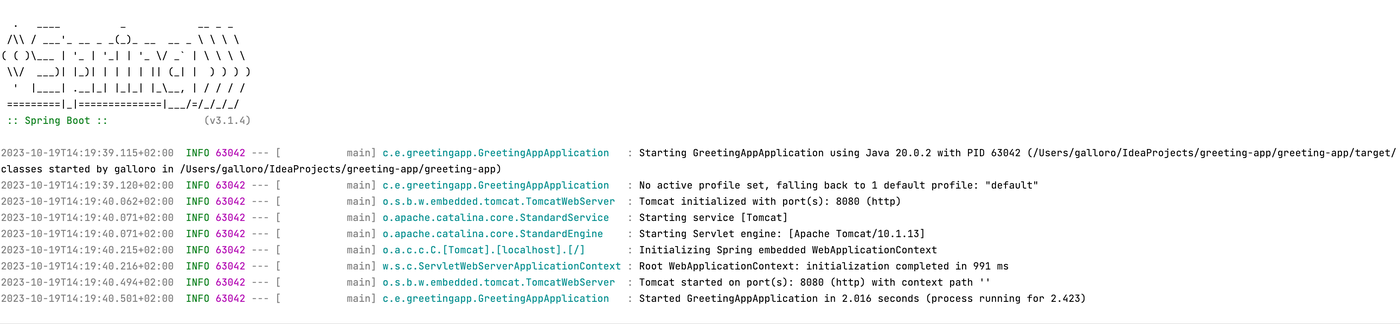
12. By default, the built-in Apache Tomcat server is listening on port 8080. Open your web browser and go to http://localhost:8080/hello
. If the code generated by Gemini works, you should see your application respond with Hello World!
13. You can also provide your name in your web request to let the application know how to greet you properly. For example you can try http://localhost:8080/hello?name=John
14. Go back to IntelliJ and type Ctrl+C in the terminal to stop the application
15. Now let’s try to improve this app a bit: create an index.html file inside the resources/static folder
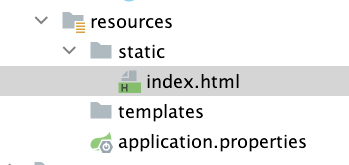
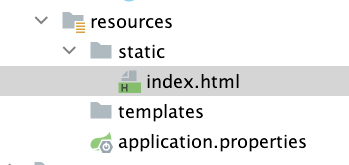
16. At the top of the file, type the following comment:
17. Hit Enter and then, to generate code, press Alt+\ (Windows/Linux) or Option+\ (macOS). Alternatively you can click on the Gemini icon near the comment line
18. You should get code generated in the form of ghost text, functionally similar to the following
19. If you get the same code as above, hit Tab to accept the suggestion. If code is different you can evaluate if it will work for your goal, decide to make some changes or use the example code above
20. Run your application again with:
21. Now, since you added an index.html file, access your application at the root from your browser: http://localhost:8080/
. You should see the Greetings Form
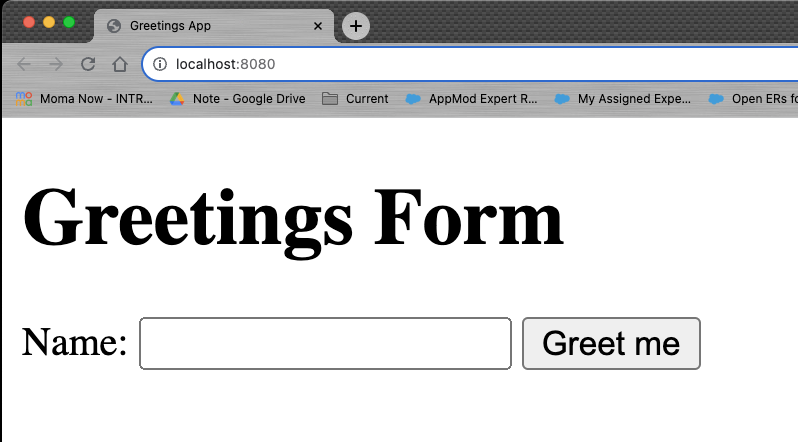
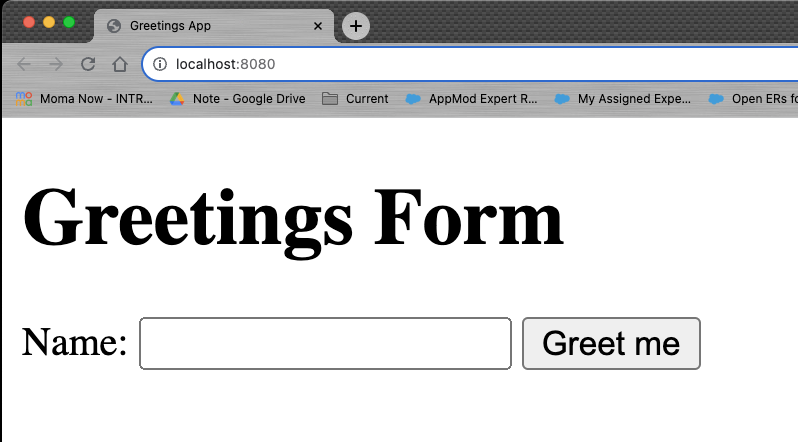
22. Type your name and click Greet me, you will be greeted
23. Go back to IntelliJ, type Ctrl+C in the terminal to stop the application
Add unit tests to your application
1. Under src/test/java/com.example.greetingapp create a new file called HomeControllerTest.java
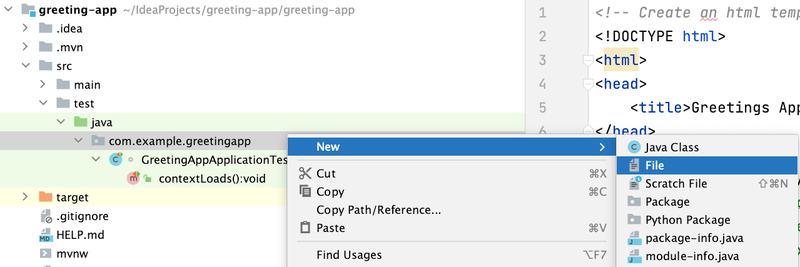
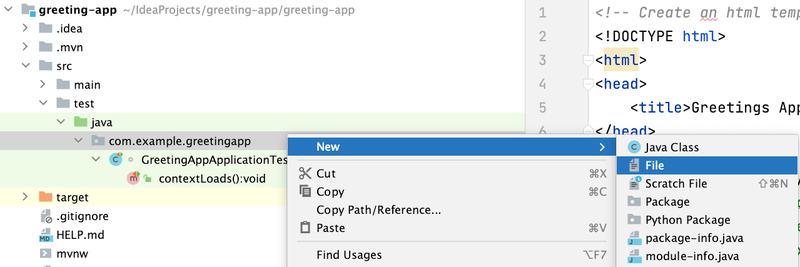
2. At the top of the file, type the following comment:
3. Hit Enter and then, to generate code, press Alt+\ (Windows/Linux) or Option+\ (macOS). Alternatively you can click on the Gemini icon near the comment line
4. You should get code generated in the form of ghost text, functionally similar to the following, testing both for the default "Hello World!" string than for a custom value of the name variable:
5. If you get the same code as above, hit Tab to accept the suggestion. If code is different you can evaluate if it will cover your test cases, decide to make some changes or use the example code above
6. In the terminal, run
7. Test should run successfully
8. Now replace the word “Hello” with “Hi” in the /hello method in your HomeController.java file and run tests again, tests should fail since the application is not responding with the expected string
Conclusions
Reading and following the instructions in this article you learned how to use Gemini Code Assist in IntelliJ to generate Java code, HTML and unit tests to start from an empty Spring Boot application and get to a working “Greeting App”.
You can learn more about Gemini capabilities for developers in: